The CHECK constraint is used to limit the value range that can be placed in a column. If the condition evaluates to FALSE, the data is rejected. This ensures data validity by enforcing rules at the column or table level.
SQL Check Syntax
SQL
x
1
CREATE TABLE table_name (
2
column_name datatype CHECK (condition)
3
);
You can also define a CHECK constraint on multiple columns:
SQL
1
1
CREATE TABLE table_name (
2
column1 datatype,
3
column2 datatype,
4
CHECK (column1 > column2)
5
);
SQL Check Example
SQL Check on Create Table
This query applies a CHECK constraint while creating the table, ensuring that the date of birth (dob) is not in the future:
SQL
1
1
CREATE TABLE PatientsWithDOBCheck (
2
patient_id INTEGER PRIMARY KEY,
3
first_name VARCHAR(50) NOT NULL,
4
last_name VARCHAR(50) NOT NULL,
5
dob DATE CHECK (dob <= DATE('now')),
6
gender CHAR(1) NOT NULL,
7
contact_number VARCHAR(15)
8
);
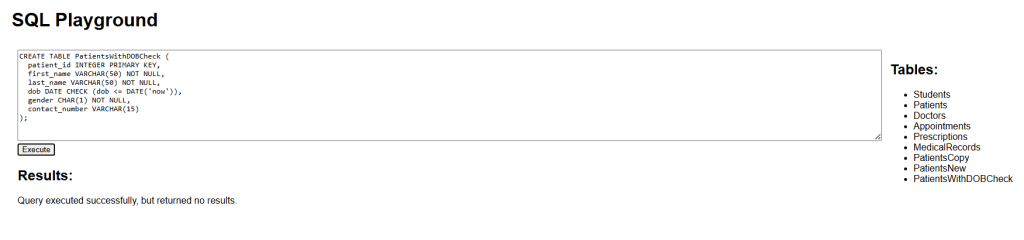
SQL Check on Alter Table
SQLite does not support adding CHECK constraints using ALTER TABLE. The only workaround is to recreate the table with the new CHECK constraint and copy the data from the original table.
Steps:
- Create a new table with the desired CHECK constraint:
SQL
1
1
CREATE TABLE PatientsNew (
2
patient_id INTEGER PRIMARY KEY,
3
first_name VARCHAR(50) NOT NULL,
4
last_name VARCHAR(50) NOT NULL,
5
dob DATE NOT NULL,
6
gender CHAR(1) NOT NULL CHECK (gender IN ('M', 'F')),
7
contact_number VARCHAR(15)
8
);
- Copy data from the existing table:
SQL
1
1
INSERT INTO PatientsNew (patient_id, first_name, last_name, dob, gender, contact_number)
2
SELECT patient_id, first_name, last_name, dob, gender, contact_number FROM Patients;
- Drop the old table (optional — do with caution):
SQL
1
1
DROP TABLE Patients;
- Rename the new table:
SQL
1
1
ALTER TABLE PatientsNew RENAME TO Patients;
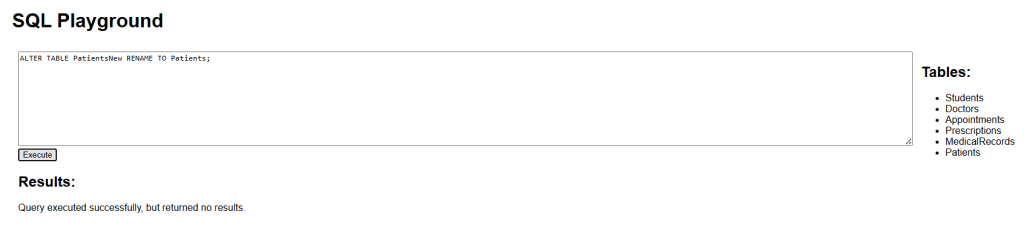
SQL Check Drop a Check Constraint
SQLite does not support dropping a CHECK constraint directly.
If needed, you must:
- Create a new table without the constraint
- Move the data over
- Drop the old table
- Rename the new one