A while
loop allows you to execute a block of code as long as a specified condition is True
. This is useful for scenarios where you need to repeat an action until a certain condition changes.
Basic While Loop
The basic syntax of a while
loop is as follows:
Explanation of Code:
The while
loop will continue to execute the indented code block as long as the condition remains True
.
while condition: # Code to be executed
Explanation of Code:
In this example, the loop will print the value of count and increment it by 1 each time, until count reaches 5.
count = 0 while count < 5: print("Count:", count) count += 1
While Loop with Else
You can also include an else
block with a while
loop. The else
block will be executed when the condition becomes False
.
Explanation of Code:
The else
block runs after the while
loop completes normally.
count = 0 while count < 5: print("Count:", count) count += 1 else: print("Count reached 5")
Infinite While Loop
An infinite loop occurs when the condition of a while
loop never becomes False
. This should generally be avoided unless intentionally used (e.g., in servers).
Explanation of Code:
An infinite loop will continue to run indefinitely unless externally stopped.
while True: print("This is an infinite loop. Press Ctrl+C to stop.")
Breaking out of a While Loop
You can use the break
statement to exit a while
loop prematurely, even if the condition is still True
.
Explanation of Code:
The break
statement immediately terminates the loop.
count = 0 while count < 10: print("Count:", count) if count == 5: break count += 1
Skipping Iterations in a While Loop
The continue
statement skips the current iteration and moves to the next iteration of the loop.
Explanation of Code:
The continue
statement allows you to skip specific iterations based on a condition.
count = 0 while count < 10: count += 1 if count % 2 == 0: continue print("Odd Count:", count)
Python While Loops Example Code
Explanation pf Code:
This program uses while
loops to print numbers, break out of the loop prematurely, and skip certain iterations.
# Basic while loop count = 0 while count < 5: print("Count:", count) count += 1 # While loop with else count = 0 while count < 5: print("Count:", count) count += 1 else: print("Count reached 5") # Infinite while loop # Uncomment the lines below to run an infinite loop # while True: # print("This is an infinite loop. Press Ctrl+C to stop.") # Breaking out of a while loop count = 0 while count < 10: print("Count:", count) if count == 5: break count += 1 # Skipping iterations in a while loop count = 0 while count < 10: count += 1 if count % 2 == 0: continue print("Odd Count:", count)
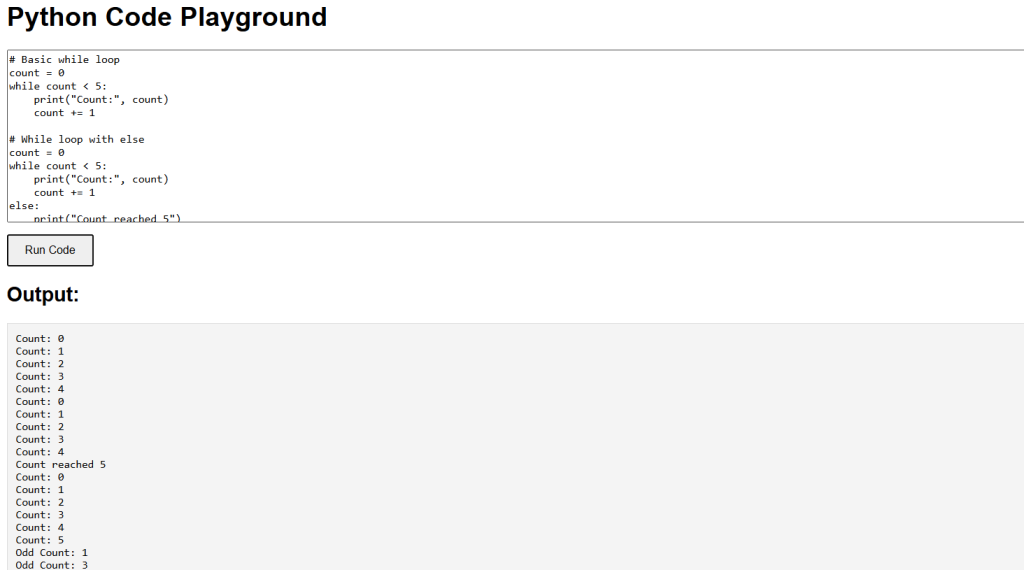