Variables are used to store data that can be referenced and manipulated in a program. In Python, variables are created when you assign a value to them. Unlike some other programming languages, Python does not require you to declare the type of variable. The type is determined dynamically at runtime.
Declaring Variables
In Python, you can create a variable by assigning a value to a name.
Explanation of Code:
In the example above, x
is an integer variable with a value of 5
, and y
is a string variable with a value of "Hello, World!"
.
x = 5
y = "Hello, World!"
Variable Naming Rules
There are some rules and conventions for naming variables in Python:
- Variable names must start with a letter (a-z, A-Z) or an underscore (_).
- The rest of the name can contain letters, numbers (0-9), and underscores.
- Variable names are case-sensitive (e.g.,
age
,Age
, andAGE
are different variables). - Avoid using Python reserved words as variable names (e.g.,
if
,for
,while
, etc.).
Example of Valid and Invalid Variable Names:
# Valid variable names
name = "Alice"
_age = 25
number_of_students = 30
# Invalid variable names
2name = "Alice" # Cannot start with a number
name! = "Alice" # Cannot contain special characters
Assigning Multiple Values
Python allows you to assign values to multiple variables in one line.
Explanation of Code:
In this example, a
is assigned a value of 1
, b
is assigned a value of 2
, and c
is assigned a value of 3
.
a, b, c = 1, 2, 3
Assigning the Same Value to Multiple Variables
You can also assign the same value to multiple variables in one line.
Explanation of Code:
In this example, x
, y
, and z
are all assigned a value of 0
.
x = y = z = 0
Dynamic Typing
Python is a dynamically typed language, which means that the type of a variable can change during the execution of a program.
Explanation of Code:
Initially, x
is an integer with a value of 5
. Later, x
is assigned a string value "Five"
, and its type changes accordingly.
x = 5 # x is an integer
x = "Five" # Now x is a string
Python Variables Example Code
Explanation of Code:
This program assigns values to the variables name
, age
, and height
, prints them, updates the values of age
and height
, and then prints the updated values.
# Assign values to variables
name = "Alice"
age = 25
height = 5.6
# Print variables
print("Name:", name)
print("Age:", age)
print("Height:", height)
# Update variables
age = 26 # Changing the value of age
height = height + 0.1 # Incrementing height
# Print updated variables
print("Updated Age:", age)
print("Updated Height:", height)
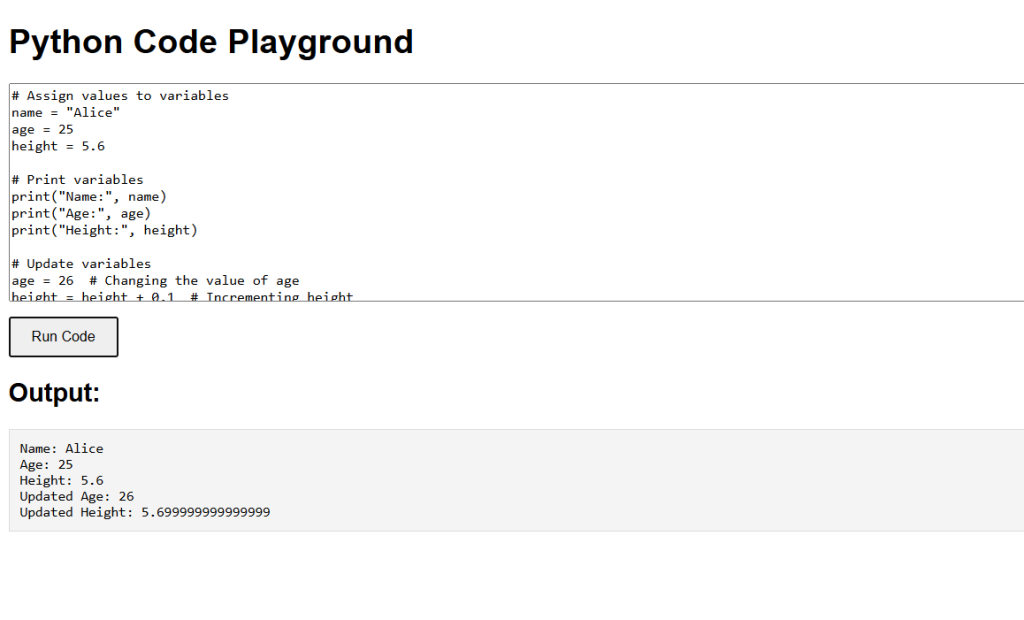