Tuples are ordered, immutable collections of items. Once a tuple is created, its elements cannot be changed. Tuples are often used to store related pieces of information and can contain items of different data types.
Creating Tuples
You can create a tuple by placing comma-separated values inside parentheses.
Explanation: Tuples can store multiple items in a single variable. They can include different data types such as strings, integers, floats, and even other tuples.
# Creating a tuple of strings fruits = ("apple", "banana", "cherry") # Creating a tuple of integers numbers = (1, 2, 3, 4, 5) # Creating a tuple with mixed data types mixed_tuple = (1, "Hello", 3.14, True)
Accessing Tuple Items
You can access individual items in a tuple using indexing. Python uses zero-based indexing.
Explanation: The index 0
accesses the first item in the tuple, while the index -1
accesses the last item.
# Accessing tuple items print(fruits[0]) # Output: apple print(fruits[-1]) # Output: cherry
Immutable Nature of Tuples
Tuples are immutable, meaning you cannot change their items after creation.
Explanation: Attempting to change an item in a tuple will result in an error.
# Trying to modify a tuple (this will raise an error) # fruits[1] = "blueberry"
Tuple Methods
Tuples have built-in methods like count()
and index()
.
- count(): Returns the number of times a specified value appears in the tuple.
- index(): Returns the index of the first occurrence of a specified value.
# Tuple methods count_of_apples = fruits.count("apple") index_of_banana = fruits.index("banana") print("Count of apples:", count_of_apples) # Output: 1 print("Index of banana:", index_of_banana) # Output: 1
Using Tuples for Multiple Assignments
Tuples are commonly used to assign multiple variables at once.
Explanation: You can unpack a tuple into multiple variables.
# Multiple assignment with tuples coordinates = (10, 20) x, y = coordinates print("x:", x) # Output: 10 print("y:", y) # Output: 20
Python Tuples Example Code
Explanation: This program creates a tuple of fruits and demonstrates accessing items, using tuple methods, and multiple assignments.
# Creating a tuple fruits = ("apple", "banana", "cherry") # Accessing tuple items print(fruits[0]) # Output: apple print(fruits[-1]) # Output: cherry # Tuple methods count_of_apples = fruits.count("apple") index_of_banana = fruits.index("banana") print("Count of apples:", count_of_apples) # Output: 1 print("Index of banana:", index_of_banana) # Output: 1 # Multiple assignment with tuples coordinates = (10, 20) x, y = coordinates print("x:", x) # Output: 10 print("y:", y) # Output: 20
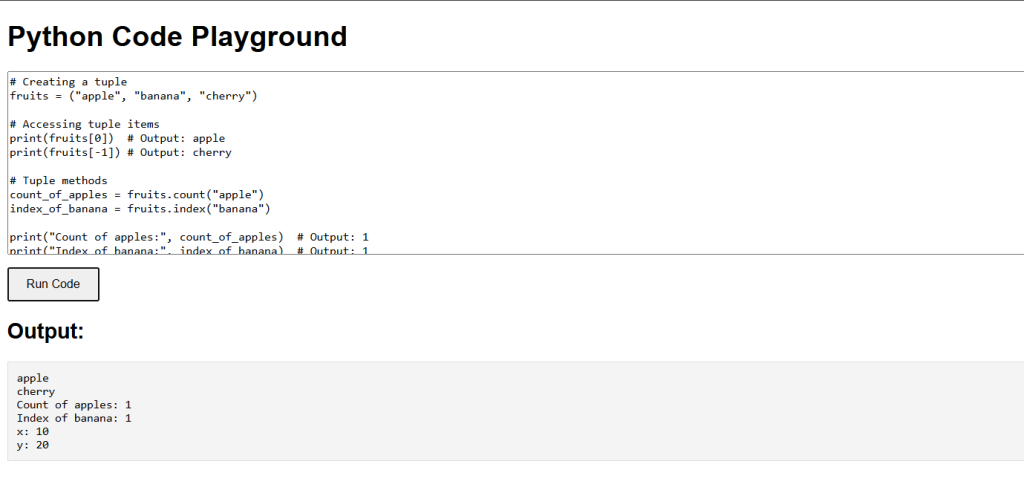