Sets are unordered collections of unique items. They are useful for storing items where duplicates are not allowed. Sets are mutable, meaning you can add or remove items after creation.
Creating Sets
You can create a set by placing comma-separated values inside curly braces {}
or by using the set()
function.
Explanation of Code:
Sets can store multiple items, and they automatically remove duplicate entries.
# Creating a set using curly braces
fruits = {"apple", "banana", "cherry", "apple"}
# Creating a set using the set() function
numbers = set([1, 2, 3, 4, 5, 5])
print(fruits) # Output: {'apple', 'banana', 'cherry'}
print(numbers) # Output: {1, 2, 3, 4, 5}
Accessing Set Items
Since sets are unordered, you cannot access items by index. However, you can loop through the set items using a for
loop.
Explanation of Code:
You can iterate through the set to access its items.
# Looping through set items
for fruit in fruits:
print(fruit)
Adding Items to a Set
You can add items to a set using the add()
method or the update()
method for adding multiple items.
add()
adds a single item to the set.update()
adds multiple items to the set.
# Adding items to a set
fruits.add("orange")
fruits.update(["mango", "grapes"])
print(fruits) # Output: {'apple', 'banana', 'cherry', 'orange', 'mango', 'grapes'}
Removing Items from a Set
You can remove items from a set using the remove()
method, the discard()
method, or the pop()
method.
remove()
removes a specified item and raises an error if the item does not exist.discard()
removes a specified item without raising an error if the item does not exist.pop()
removes and returns an arbitrary item from the set.
# Removing items from a set
fruits.remove("banana")
fruits.discard("cherry")
removed_item = fruits.pop()
print(fruits) # Output: Remaining items in the set
print(removed_item) # Output: The item that was removed
Set Operations
Python provides several built-in set operations, including union, intersection, difference, and symmetric difference.
- union(): Returns a set containing all items from both sets.
- intersection(): Returns a set containing only the items that are present in both sets.
- difference(): Returns a set containing items that are only in the first set and not in the second set.
- symmetric_difference(): Returns a set containing items that are in either set, but not in both.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
intersection_set = set1.intersection(set2)
difference_set = set1.difference(set2)
symmetric_difference_set = set1.symmetric_difference(set2)
print("Union:", union_set) # Output: {1, 2, 3, 4, 5}
print("Intersection:", intersection_set) # Output: {3}
print("Difference:", difference_set) # Output: {1, 2}
print("Symmetric Difference:", symmetric_difference_set) # Output: {1, 2, 4, 5}
Python Sets Example Code
Explanation of Code:
This program creates a set of fruits and demonstrates adding, removing items, and performing set operations.
# Creating a set
fruits = {"apple", "banana", "cherry"}
# Adding items to a set
fruits.add("orange")
fruits.update(["mango", "grapes"])
print(fruits) # Output: {'apple', 'banana', 'cherry', 'orange', 'mango', 'grapes'}
# Removing items from a set
fruits.remove("banana")
fruits.discard("cherry")
removed_item = fruits.pop()
print(fruits) # Output: Remaining items in the set
print(removed_item) # Output: The item that was removed
# Set operations
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
intersection_set = set1.intersection(set2)
difference_set = set1.difference(set2)
symmetric_difference_set = set1.symmetric_difference(set2)
print("Union:", union_set) # Output: {1, 2, 3, 4, 5}
print("Intersection:", intersection_set) # Output: {3}
print("Difference:", difference_set) # Output: {1, 2}
print("Symmetric Difference:", symmetric_difference_set) # Output: {1, 2, 4, 5}
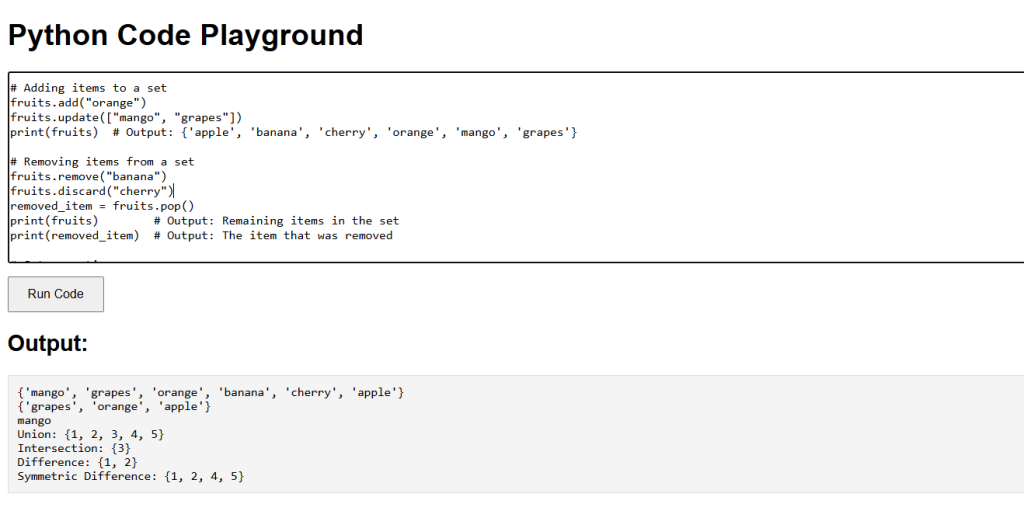