Python supports three main types of numbers: integers, floating-point numbers, and complex numbers. Each type has its own characteristics and uses. Python also provides various built-in functions and operators to perform mathematical operations.
Integer (int)
Integers are whole numbers, both positive and negative, without a decimal point.
Explanation of Code:
x
is a positive integer, and y
is a negative integer.
x = 10 y = -3
Floating-Point (float)
Floating-point numbers are real numbers with a decimal point. They can also be represented in scientific notation.
Explanation of Code:
a
and b
are floating-point numbers, while c
is a floating-point number represented in scientific notation.
a = 3.14 b = -2.5 c = 1.5e2 # Equivalent to 1.5 * 10^2 or 150.0
Complex (complex)
Complex numbers have a real part and an imaginary part, and they are written in the form a + bj
.
Explanation of Code:
d is a complex number with a real part 2
and an imaginary part 3j
. e
is another complex number created using the complex()
function.
d = 2 + 3j e = complex(1, -1)
Basic Mathematical Operations
1. Addition (+)
pythonresult = x + a # Adds an integer and a float
2. Subtraction (-)
pythonresult = x - y # Subtracts one integer from another
3. Multiplication (*)
result = a * b # Multiplies two floats
4. Division (/)
result = x / y # Divides one integer by another
5. Floor Division (//)
result = x // y # Performs floor division
6. Modulus (%)
result = x % y # Returns the remainder of the division
7. Exponentiation(**)
result = x ** 2 # Raises x to the power of 2
Built-in Mathematical Functions
Python provides several built-in functions for mathematical operations.
1. abs()
absolute_value = abs(-5) # Returns 5
2. round()
rounded_value = round(3.14159, 2) # Returns 3.14
3. pow()
power = pow(2, 3) # Returns 8
4. max() and min()
maximum = max(1, 2, 3, 4, 5) # Returns 5 minimum = min(1, 2, 3, 4, 5) # Returns 1
Python Numbers Example Code
Explanation of Code:
This program declares several variables and performs various mathematical operations using both operators and built-in functions. The results are printed to the console.
# Declare variables x = 10 y = 3 a = 3.14 b = 2.0 # Addition print("Addition:", x + y) # Subtraction print("Subtraction:", x - y) # Multiplication print("Multiplication:", x * y) # Division print("Division:", x / y) # Floor Division print("Floor Division:", x // y) # Modulus print("Modulus:", x % y) # Exponentiation print("Exponentiation:", x ** y) # Absolute Value print("Absolute Value:", abs(-x)) # Round print("Round:", round(a, 1)) # Power print("Power:", pow(x, y)) # Max and Min print("Max:", max(x, y, a, b)) print("Min:", min(x, y, a, b))
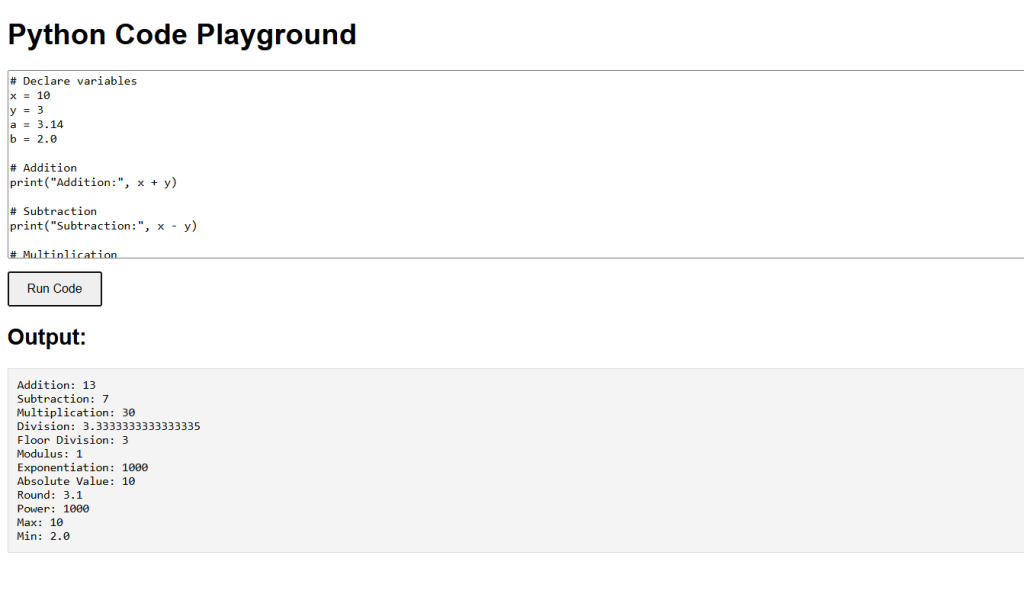