Python provides a wide range of built-in mathematical functions and operators. Additionally, the math
module offers many useful mathematical functions beyond the basic operations.
Basic Mathematical Operations
Python supports basic arithmetic operations such as addition, subtraction, multiplication, and division.
+
: Addition-
: Subtraction*
: Multiplication/
: Division
Python
x
8
1
# Basic arithmetic operations
2
a = 10
3
b = 5
4
5
print("Addition:", a + b) # Output: 15
6
print("Subtraction:", a - b) # Output: 5
7
print("Multiplication:", a * b) # Output: 50
8
print("Division:", a / b) # Output: 2.0
Using the math
Module
The math
module provides access to various mathematical functions and constants.
math.sqrt(x)
: Returns the square root ofx
.math.pow(x, y)
: Returnsx
raised to the power ofy
.math.pi
: The mathematical constant π (pi).math.e
: The mathematical constant e (Euler’s number).
Python
1
7
1
import math
2
3
# Using math module functions and constants
4
print("Square root of 16:", math.sqrt(16)) # Output: 4.0
5
print("2 raised to the power of 3:", math.pow(2, 3)) # Output: 8.0
6
print("Value of pi:", math.pi) # Output: 3.141592653589793
7
print("Value of e:", math.e) # Output: 2.718281828459045
Trigonometric Functions
The math
module includes trigonometric functions for performing calculations involving angles.
math.sin(x)
: Returns the sine ofx
(in radians).math.cos(x)
: Returns the cosine ofx
(in radians).math.tan(x)
: Returns the tangent ofx
(in radians).math.radians(x)
: Converts degrees to radians.math.degrees(x)
: Converts radians to degrees.
Python
1
11
11
1
import math
2
3
# Using trigonometric functions
4
angle_degrees = 45
5
angle_radians = math.radians(angle_degrees)
6
7
print("Sine of 45 degrees:", math.sin(angle_radians)) # Output: 0.7071067811865476
8
print("Cosine of 45 degrees:", math.cos(angle_radians)) # Output: 0.7071067811865476
9
print("Tangent of 45 degrees:", math.tan(angle_radians)) # Output: 1.0
10
print("45 degrees in radians:", angle_radians) # Output: 0.7853981633974483
11
print("0.785 radians in degrees:", math.degrees(angle_radians)) # Output: 45.0
Logarithmic Functions
The math
module also includes logarithmic functions for performing log calculations.
math.log(x)
: Returns the natural logarithm ofx
.math.log10(x)
: Returns the base-10 logarithm ofx
.
Python
1
5
1
import math
2
3
# Using logarithmic functions
4
print("Natural log of 10:", math.log(10)) # Output: 2.302585092994046
5
print("Base-10 log of 100:", math.log10(100)) # Output: 2.0
Python Math Example Code
- Basic arithmetic operations: Demonstrates addition, subtraction, multiplication, and division.
- Using the
math
module: Shows the use of functions and constants in themath
module. - Trigonometric functions: Uses trigonometric functions for angle calculations.
- Logarithmic functions: Performs logarithmic calculations.
Python
1
30
30
1
import math
2
3
# Basic arithmetic operations
4
a = 10
5
b = 5
6
7
print("Addition:", a + b) # Output: 15
8
print("Subtraction:", a - b) # Output: 5
9
print("Multiplication:", a * b) # Output: 50
10
print("Division:", a / b) # Output: 2.0
11
12
# Using math module functions and constants
13
print("Square root of 16:", math.sqrt(16)) # Output: 4.0
14
print("2 raised to the power of 3:", math.pow(2, 3)) # Output: 8.0
15
print("Value of pi:", math.pi) # Output: 3.141592653589793
16
print("Value of e:", math.e) # Output: 2.718281828459045
17
18
# Using trigonometric functions
19
angle_degrees = 45
20
angle_radians = math.radians(angle_degrees)
21
22
print("Sine of 45 degrees:", math.sin(angle_radians)) # Output: 0.7071067811865476
23
print("Cosine of 45 degrees:", math.cos(angle_radians)) # Output: 0.7071067811865476
24
print("Tangent of 45 degrees:", math.tan(angle_radians)) # Output: 1.0
25
print("45 degrees in radians:", angle_radians) # Output: 0.7853981633974483
26
print("0.785 radians in degrees:", math.degrees(angle_radians)) # Output: 45.0
27
28
# Using logarithmic functions
29
print("Natural log of 10:", math.log(10)) # Output: 2.302585092994046
30
print("Base-10 log of 100:", math.log10(100)) # Output: 2.0
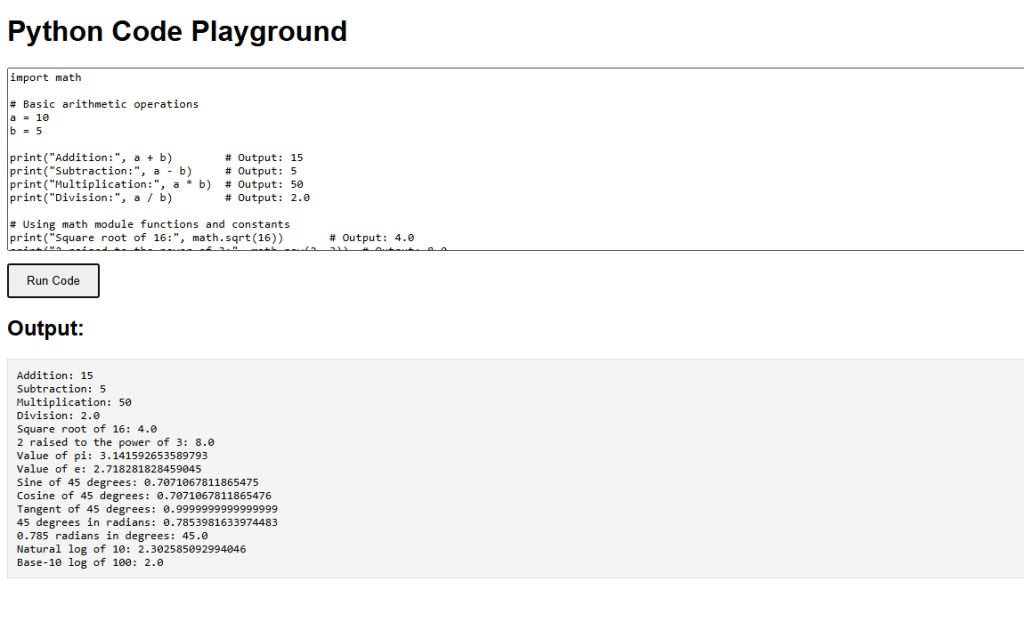