Conditional statements allow you to execute different blocks of code based on whether a condition is True
or False
. The primary conditional statements in Python are if
, elif
, and else
.
If Statement
The if
statement evaluates a condition, and if the condition is True
, it executes the indented block of code that follows.
Explanation of Code:
The if
statement is used to check a condition. If the condition is True
, the code block under the if
statement runs.
x = 10 if x > 5: print("x is greater than 5")
Elif Statement
The elif
(else if) statement checks another condition if the previous if
or elif
condition was False
.
Explanation of Code:
You can use multiple elif
statements to check additional conditions if previous conditions are not met.
x = 10 if x > 15: print("x is greater than 15") elif x > 5: print("x is greater than 5 but less than or equal to 15")
Else Statement
The else
statement executes a block of code if none of the preceding if
or elif
conditions are True
.
Explanation of Code:
The else
block runs if all previous conditions are False
.
x = 10 if x > 15: print("x is greater than 15") elif x > 5: print("x is greater than 5 but less than or equal to 15") else: print("x is less than or equal to 5")
Nested If Statements
You can nest if
, elif
, and else
statements within each other to create complex conditional logic.
Explanation of Code:
Nested if
statements allow you to check multiple conditions at different levels.
x = 10 y = 20 if x > 5: if y > 15: print("x is greater than 5 and y is greater than 15") else: print("x is greater than 5 but y is not greater than 15") else: print("x is not greater than 5")
Python If…Else Example Code
Explanation of Code:
This program uses conditional statements to determine and print messages based on the value of the variable x
.
# If statement x = 10 if x > 5: print("x is greater than 5") # If...elif...else statement if x > 15: print("x is greater than 15") elif x > 5: print("x is greater than 5 but less than or equal to 15") else: print("x is less than or equal to 5") # Nested if statements y = 20 if x > 5: if y > 15: print("x is greater than 5 and y is greater than 15") else: print("x is greater than 5 but y is not greater than 15") else: print("x is not greater than 5")
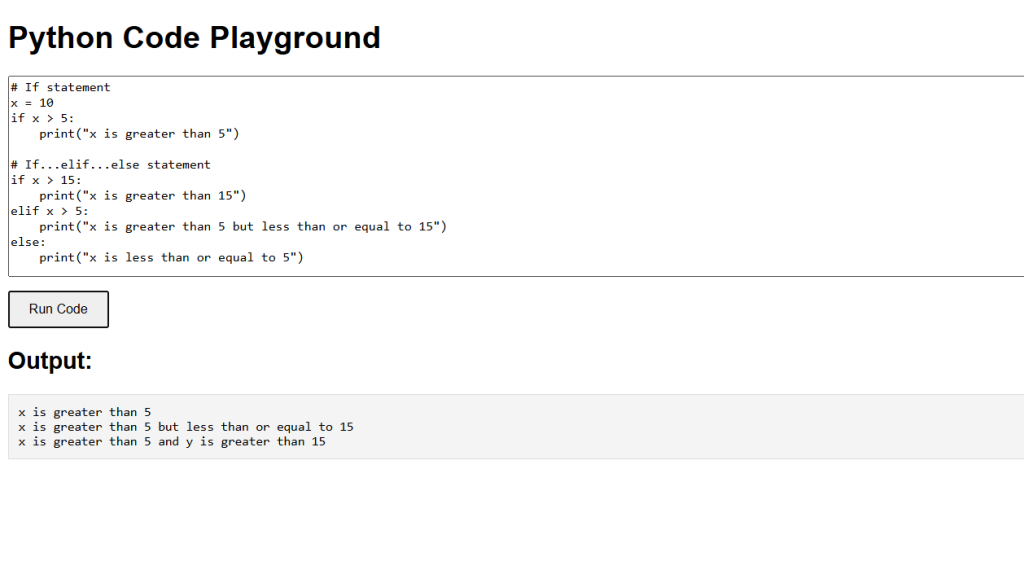