Python provides a built-in module called datetime
for working with dates and times. This module allows you to create datetime
objects, manipulate them, and format them according to your needs.
Getting the Current Date and Time
To get the current date and time, you can use the datetime
module.
datetime.now()
: Returns the current local date and time.
Python
x
5
1
from datetime import datetime
2
3
# Get the current date and time
4
now = datetime.now()
5
print("Current date and time:", now)
Creating Date Objects
You can create a date object by using the date
class from the datetime
module.
date(year, month, day)
: Creates a date object representing the specified date.
Python
1
5
1
from datetime import date
2
3
# Create a date object
4
birthday = date(1990, 5, 15)
5
print("Birthday:", birthday)
Creating Time Objects
To create a time object, use the time
class from the datetime
module.
time(hour, minute, second)
: Creates a time object representing the specified time.
Python
1
5
1
from datetime import time
2
3
# Create a time object
4
time_of_day = time(14, 30, 15)
5
print("Time of day:", time_of_day)
Formatting Dates and Times
You can format date and time objects using the strftime
method, which converts them into a string representation based on a specified format.
strftime(format)
: Returns a string representation of the date and time object, formatted according to the specified format.
Python
1
8
1
from datetime import datetime
2
3
# Get the current date and time
4
now = datetime.now()
5
6
# Format the date and time
7
formatted_date_time = now.strftime("%Y-%m-%d %H:%M:%S")
8
print("Formatted date and time:", formatted_date_time)
Parsing Dates and Times
You can parse a string representation of a date and time into a datetime
object using the strptime
method.
strptime(date_string, format)
: Parses a string representation of a date and time into adatetime
object based on the specified format.
Python
1
6
1
from datetime import datetime
2
3
# Parse a string into a datetime object
4
date_string = "2023-11-25 11:00:00"
5
parsed_date_time = datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S")
6
print("Parsed date and time:", parsed_date_time)
Performing Date Arithmetic
You can perform arithmetic operations on datetime
objects using the timedelta
class.
timedelta(days, seconds, minutes)
: Represents a duration for performing date arithmetic.
Python
1
8
1
from datetime import datetime, timedelta
2
3
# Get the current date and time
4
now = datetime.now()
5
6
# Add 10 days to the current date
7
future_date = now + timedelta(days=10)
8
print("Date after 10 days:", future_date)
Python Dates Example Code
- Get current date and time: Uses
datetime.now()
to get the current date and time. - Create date and time objects: Uses
date
andtime
classes to create specific date and time objects. - Format date and time: Uses
strftime
to format date and time into a string. - Parse date and time: Uses
strptime
to parse a string into adatetime
object. - Date arithmetic: Uses
timedelta
to perform arithmetic operations on dates.
Python
1
26
26
1
from datetime import datetime, date, time, timedelta
2
3
# Get the current date and time
4
now = datetime.now()
5
print("Current date and time:", now)
6
7
# Create a date object
8
birthday = date(1990, 5, 15)
9
print("Birthday:", birthday)
10
11
# Create a time object
12
time_of_day = time(14, 30, 15)
13
print("Time of day:", time_of_day)
14
15
# Format the date and time
16
formatted_date_time = now.strftime("%Y-%m-%d %H:%M:%S")
17
print("Formatted date and time:", formatted_date_time)
18
19
# Parse a string into a datetime object
20
date_string = "2023-11-25 11:00:00"
21
parsed_date_time = datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S")
22
print("Parsed date and time:", parsed_date_time)
23
24
# Add 10 days to the current date
25
future_date = now + timedelta(days=10)
26
print("Date after 10 days:", future_date)
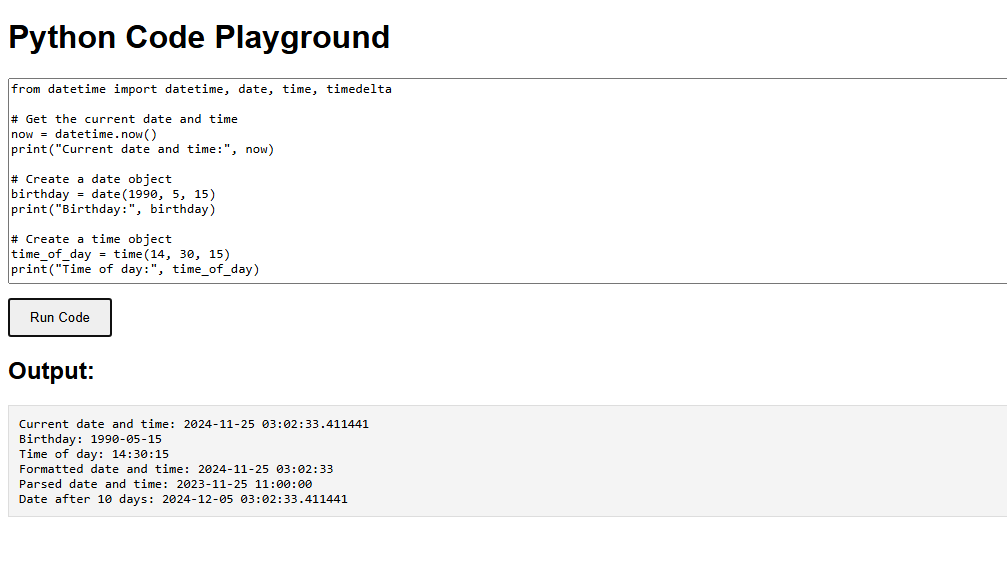