Data types specify the type of data that a variable can hold. Python has various built-in data types that allow you to work with different kinds of data effectively.
Common Data Types in Python
- Numeric Types: Integers, Floating-Point Numbers, and Complex Numbers
- Sequence Types: Strings, Lists, and Tuples
- Mapping Type: Dictionaries
- Set Types: Sets and Frozensets
- Boolean Type: Booleans
- Binary Types: Bytes, Byte Arrays, and Memory Views
Numeric Types
1. Integers
Integers are whole numbers, both positive and negative, without a fractional part.
x = 10
y = -5
2. Floating-Point Numbers
Floating-point numbers are real numbers with a decimal point.
a = 3.14
b = -2.5
3. Complex Numbers
Complex numbers are written with a real part and an imaginary part.
c = 2 + 3j
d = complex(1, -1)
Sequence Types
1. Strings
Strings are sequences of characters enclosed in single or double quotes.
text = "Hello, World!"
2. Lists
Lists are ordered, mutable collections of items.
fruits = ["apple", "banana", "cherry"]
3. Tuples
Tuples are ordered, immutable collections of items.
coordinates = (10.0, 20.0)
Mapping Type
1. Dictionaries
Dictionaries are unordered collections of key-value pairs.
student = {"name": "Alice", "age": 21, "course": "Computer Science"}
Set Types
1. Sets
Sets are unordered collections of unique items.
fruits = {"apple", "banana", "cherry"}
2. Frozensets
Frozensets are immutable sets.
frozen_fruits = frozenset({"apple", "banana", "cherry"})
Boolean Type
1. Booleans
Booleans represent True
or False
values.
is_active = True
is_student = False
Binary Types
1. Bytes
Bytes represent sequences of byte (8-bit) values.
byte_data = b"Hello"
2. Byte Arrays
Byte arrays are mutable sequences of byte values.
byte_array = bytearray(5)
3. Memory Views
Memory views allow you to access the internal data of an object that supports the buffer protocol.
memory_view = memoryview(byte_array)
Python Data Types Example Code
This example demonstrates how to define and print variables of different data types in Python.
# Numeric Types
integer_number = 10
float_number = 3.14
complex_number = 2 + 3j
# Sequence Types
string_text = "Hello, World!"
list_fruits = ["apple", "banana", "cherry"]
tuple_coordinates = (10.0, 20.0)
# Mapping Type
dict_student = {"name": "Alice", "age": 21, "course": "Computer Science"}
# Set Types
set_fruits = {"apple", "banana", "cherry"}
frozenset_fruits = frozenset({"apple", "banana", "cherry"})
# Boolean Type
bool_is_active = True
bool_is_student = False
# Binary Types
bytes_data = b"Hello"
bytearray_data = bytearray(5)
memoryview_data = memoryview(bytearray_data)
# Print Variables
print("Integer:", integer_number)
print("Float:", float_number)
print("Complex:", complex_number)
print("String:", string_text)
print("List:", list_fruits)
print("Tuple:", tuple_coordinates)
print("Dictionary:", dict_student)
print("Set:", set_fruits)
print("Frozenset:", frozenset_fruits)
print("Boolean:", bool_is_active, bool_is_student)
print("Bytes:", bytes_data)
print("Bytearray:", bytearray_data)
print("Memoryview:", memoryview_data)
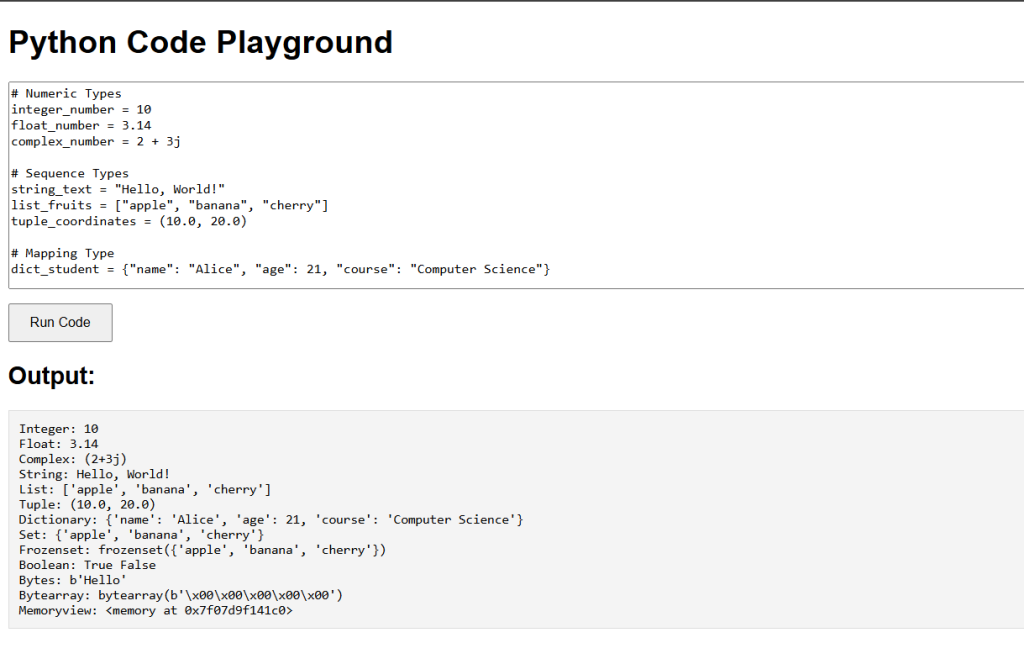