An iterator is an object that contains a countable number of values. In Python, an iterator is an object which implements the iterator protocol, consisting of the methods __iter__()
and __next__()
.
Creating an Iterator
You can create an iterator from any iterable object, such as a list, tuple, or string, by using the iter()
function.
Explanation of Code:
In this example, my_list
is an iterable, and my_iterator
is an iterator created from my_list
. The next()
function is used to get the next item from the iterator.
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
Using Iterators in a Loop
Iterators are often used in loops to iterate through elements of a collection.
Explanation of Code:
You can use a for
loop to automatically handle the iteration process. This loop will continue to print each item from the iterator until all items have been exhausted.
for item in my_iterator:
print(item)
Creating a Custom Iterator
You can create a custom iterator by defining a class that implements the __iter__()
and __next__()
methods.
Explanation of Code:
In this example, MyNumbers
is a custom iterator that returns numbers from 1 to 5. The __iter__()
method initializes the iterator and the __next__()
method returns the next value.
class MyNumbers:
def __iter__(self):
self.a = 1
return self
def __next__(self):
if self.a <= 5:
x = self.a
self.a += 1
return x
else:
raise StopIteration
my_numbers = MyNumbers()
my_iterator = iter(my_numbers)
for number in my_iterator:
print(number)
Python Iterators Example Code
Explanation of Code:
This program shows how to create an iterator from a list, use it in a loop, and create a custom iterator.
# Creating an iterator from a list
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
# Using an iterator in a loop
for item in my_iterator:
print(item)
# Creating a custom iterator
class MyNumbers:
def __iter__(self):
self.a = 1
return self
def __next__(self):
if self.a <= 5:
x = self.a
self.a += 1
return x
else:
raise StopIteration
my_numbers = MyNumbers()
my_iterator = iter(my_numbers)
for number in my_iterator:
print(number)
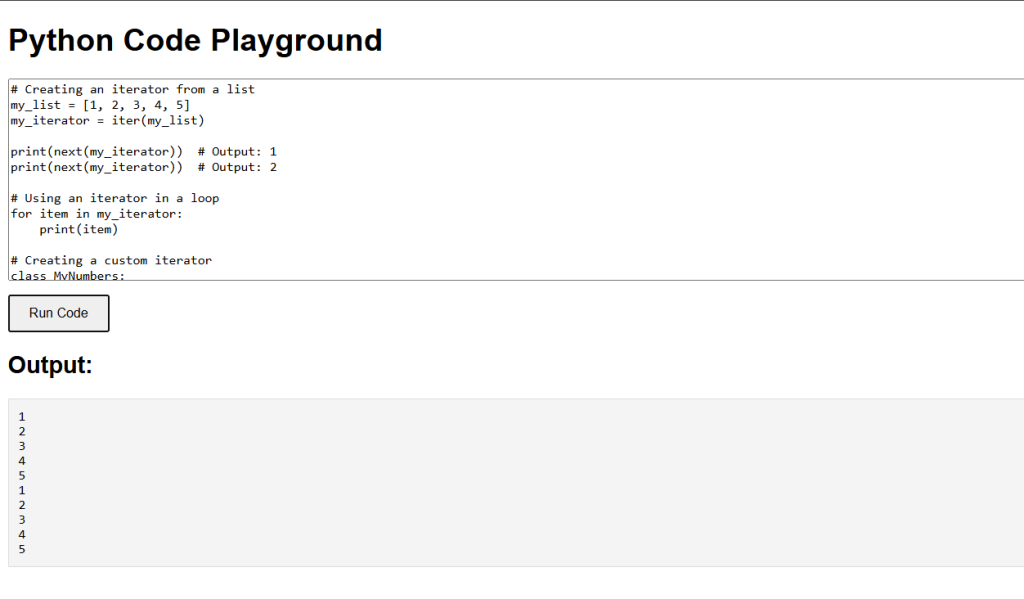