A for
loop allows you to iterate over a sequence of elements, such as a list, tuple, string, or range. It is useful for performing repetitive tasks on each item in a sequence.
Basic For Loop
The basic syntax of a for
loop is as follows:
Explanation of Code:
The for
loop iterates over each item in the sequence, executing the indented block of code for each item.
for item in sequence: # Code to be executed
Explanation of Code:
In this example, the loop prints each fruit in the fruits
list.
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
Looping Through a String
You can use a for
loop to iterate over each character in a string.
Explanation of Code:
The loop iterates over each character in the string and executes the code block for each character.
text = "Hello" for char in text: print(char)
Looping Through a Range
The range()
function generates a sequence of numbers, which can be used to control the number of times a loop executes.
Explanation of Code:
The range()
function creates a sequence of numbers from 0 up to (but not including) the specified end value. In this example, the loop prints numbers from 0 to 4.
for i in range(5): print(i)
Looping with an Else Clause
You can include an else
clause with a for
loop. The else
block will be executed after the loop finishes iterating over all items in the sequence.
Explanation of Code:
The else
block runs after the loop completes normally.
for i in range(5): print(i) else: print("Loop is finished")
Breaking out of a For Loop
You can use the break
statement to exit a for
loop prematurely, even if there are more items to iterate over.
Explanation of Code:
In this example, the loop prints numbers from 0 to 4 and then exits when i
is equal to 5. The break
statement immediately terminates the loop.
for i in range(10): if i == 5: break print(i)
Skipping Iterations in a For Loop
The continue
statement skips the current iteration and moves to the next iteration of the loop.
Explanation of Code:
In this example, the loop prints only odd numbers from 0 to 9. The continue
statement allows you to skip specific iterations based on a condition.
for i in range(10): if i % 2 == 0: continue print(i)
Nested For Loops
You can nest for
loops within each other to iterate over multi-dimensional data structures.
Explanation of Code:
This example prints each item in a 2D list (matrix) in a structured format. Nested for
loops allow you to iterate over each item in a multi-dimensional sequence.
matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] for row in matrix: for item in row: print(item, end=" ") print()
Python For Loops Example Code
Explanation of Code:
This program uses for
loops to iterate over lists, strings, ranges, and demonstrates breaking out of loops, skipping iterations, and nesting loops.
# Basic for loop fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit) # Looping through a string text = "Hello" for char in text: print(char) # Looping through a range for i in range(5): print(i) # Looping with an else clause for i in range(5): print(i) else: print("Loop is finished") # Breaking out of a for loop for i in range(10): if i == 5: break print(i) # Skipping iterations in a for loop for i in range(10): if i % 2 == 0: continue print(i) # Nested for loops matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] for row in matrix: for item in row: print(item, end=" ") print()
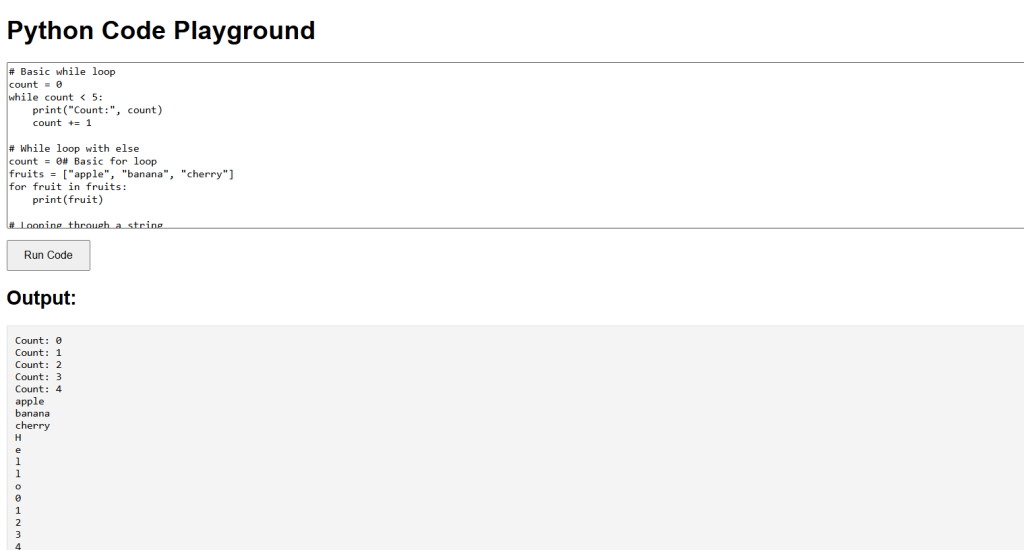