Operators are special symbols that perform operations on variables and values. Python has various types of operators, including arithmetic, comparison, logical, assignment, bitwise, and membership operators.
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations.
- Addition (+):
Adds two values.
result = 5 + 3 # Output: 8
- Subtraction (-):
Subtracts the second value from the first.
result = 10 - 6 # Output: 4
- Multiplication (*):
Multiplies two values.
result = 4 * 7 # Output: 28
- Division (/):
Divides the first value by the second.
result = 16 / 4 # Output: 4.0
- Floor Division (//):
Divides the first value by the second and returns the largest integer less than or equal to the result.
result = 17 // 3 # Output: 5
- Modulus (%):
Returns the remainder of the division.
result = 10 % 3 # Output: 1
- Exponentiation ()**:
Raises the first value to the power of the second.
result = 2 ** 3 # Output: 8
Comparison Operators
Comparison operators compare two values and return True
or False
.
- Equal to (==):
Checks if two values are equal.
result = (5 == 5) # Output: True
- Not equal to (!=):
Checks if two values are not equal.
result = (5 != 3) # Output: True
- Greater than (>):
Checks if the first value is greater than the second.
result = (7 > 3) # Output: True
- Less than (<):
Checks if the first value is less than the second.
result = (4 < 9) # Output: True
- Greater than or equal to (>=):
Checks if the first value is greater than or equal to the second.
result = (6 >= 6) # Output: True
- Less than or equal to (<=):
Checks if the first value is less than or equal to the second.
result = (8 <= 10) # Output: True
Logical Operators
Logical operators are used to combine conditional statements.
- and:
Returns True
if both conditions are true.
result = (5 > 3) and (8 > 5) # Output: True
- or:
Returns True
if at least one condition is true.
result = (5 > 3) or (8 < 5) # Output: True
- not:
Reverses the result of the condition.
result = not (5 > 3) # Output: False
Assignment Operators
Assignment operators are used to assign values to variables.
- Assign (=):
Assigns the value 5
to x
.
x = 5
- Add and assign (+=):
Adds the right operand to the left operand and assigns the result to the left operand.
x = 5
x += 3 # Equivalent to x = x + 3
- Subtract and assign (-=):
Subtracts the right operand from the left operand and assigns the result to the left operand.
x = 5
x -= 2 # Equivalent to x = x - 2
- Multiply and assign (*=):
Multiplies the left operand by the right operand and assigns the result to the left operand.
x = 5
x *= 4 # Equivalent to x = x * 4
- Divide and assign (/=):
Divides the left operand by the right operand and assigns the result to the left operand.
x = 10
x /= 2 # Equivalent to x = x / 2
- Modulus and assign (%=):
Takes the modulus using two operands and assigns the result to the left operand.
x = 10
x %= 3 # Equivalent to x = x % 3
- Exponentiation and assign (=)**:
Raises the left operand to the power of the right operand and assigns the result to the left operand.
x = 2
x **= 3 # Equivalent to x = x ** 3
Python Operators Example Code
This code demonstrates various Python operators. It includes arithmetic, comparison, logical, and assignment operations. The results are printed for each operation type.
# Arithmetic Operators
x = 5
y = 3
print("Addition:", x + y) # Output: 8
print("Subtraction:", x - y) # Output: 2
print("Multiplication:", x * y) # Output: 15
print("Division:", x / y) # Output: 1.666...
# Comparison Operators
print("Equal to:", x == y) # Output: False
print("Not equal to:", x != y) # Output: True
print("Greater than:", x > y) # Output: True
print("Less than:", x < y) # Output: False
# Logical Operators
a = True
b = False
print("Logical AND:", a and b) # Output: False
print("Logical OR:", a or b) # Output: True
print("Logical NOT:", not a) # Output: False
# Assignment Operators
x = 10
x += 5
print("Add and assign:", x) # Output: 15
x *= 2
print("Multiply and assign:", x) # Output: 30
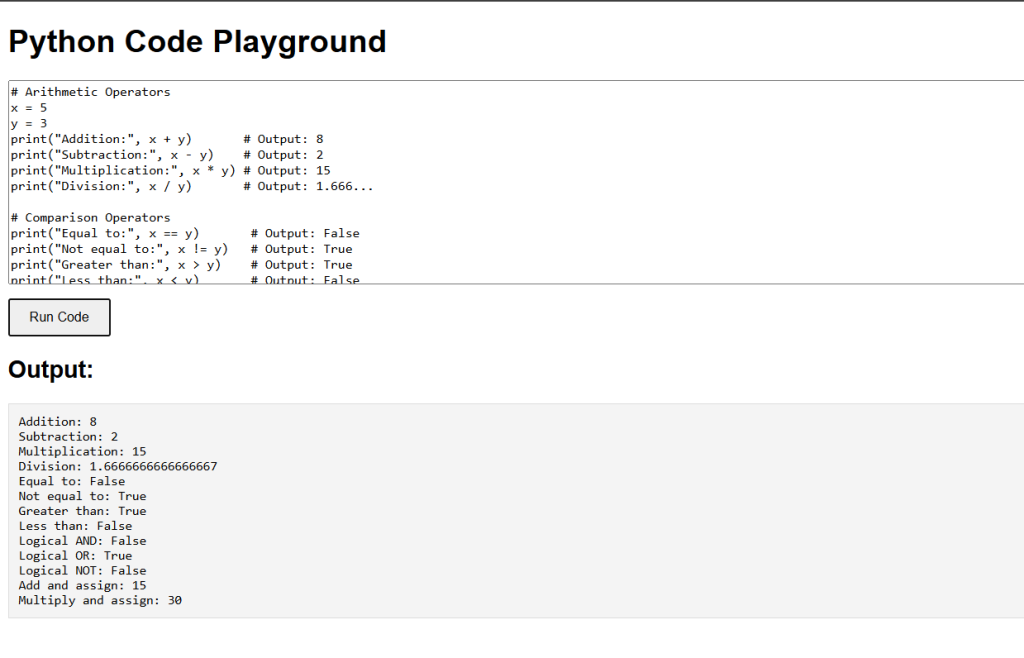