What is a String?
A string is a sequence of characters used to represent text. In JavaScript, strings are enclosed in single quotes ('
), double quotes ("
), or backticks (`
).
// Example of Strings: let singleQuote = 'Hello'; let doubleQuote = "World"; let templateLiteral = `Hello, World!`; console.log(singleQuote); // Output: Hello console.log(doubleQuote); // Output: World console.log(templateLiteral); // Output: Hello, World!
String Properties
Strings have properties that provide information about the string.
Length
The length
property returns the number of characters in a string.
let text = "JavaScript"; console.log(text.length); // Output: 10
String Methods
JavaScript provides various methods to manipulate strings.
1. charAt()
The charAt()
method returns the character at a specified index.
let text = "JavaScript"; console.log(text.charAt(0)); // Output: J console.log(text.charAt(4)); // Output: S
2. concat()
The concat()
method concatenates two or more strings.
let text1 = "Hello"; let text2 = "World"; let result = text1.concat(", ", text2, "!"); console.log(result); // Output: Hello, World!
3. includes()
The includes()
method checks if a string contains a specified substring.
let text = "JavaScript is fun"; console.log(text.includes("fun")); // Output: true console.log(text.includes("boring")); // Output: false
4. indexOf()
The indexOf()
method returns the index of the first occurrence of a specified substring.
let text = "JavaScript"; console.log(text.indexOf("S")); // Output: 4 console.log(text.indexOf("X")); // Output: -1
5. replace()
The replace()
method replaces a specified substring with another substring.
let text = "JavaScript is fun"; let newText = text.replace("fun", "awesome"); console.log(newText); // Output: JavaScript is awesome
6. slice()
The slice()
method extracts a part of a string and returns it as a new string.
let text = "JavaScript"; let part = text.slice(0, 4); console.log(part); // Output: Java
7. split()
The split()
method splits a string into an array of substrings.
let text = "Hello, World!"; let words = text.split(" "); console.log(words); // Output: ["Hello,", "World!"]
8. substring()
The substring()
method extracts characters from a string between two specified indices.
let text = "JavaScript"; let part = text.substring(4, 10); console.log(part); // Output: Script
9. toLowerCase()
and toUpperCase()
The toLowerCase()
method converts a string to lowercase, and the toUpperCase()
method converts a string to uppercase.
let text = "JavaScript"; console.log(text.toLowerCase()); // Output: javascript console.log(text.toUpperCase()); // Output: JAVASCRIPT
10. trim()
The trim()
method removes whitespace from both ends of a string.
let text = " JavaScript "; console.log(text.trim()); // Output: JavaScript
JavaScript Strings Example Code
Explanation of Code:
- String Properties: Demonstrates the use of the
length
property. - String Methods: Demonstrates the use of various string methods such as
charAt
,concat
,includes
,indexOf
,replace
,slice
,split
,substring
,toLowerCase
,toUpperCase
, andtrim
.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Strings Example</title> </head> <body> <script> let text = " JavaScript is fun! "; // String properties console.log("Length: " + text.length); // Output: Length: 20 // String methods console.log("charAt(0): " + text.charAt(0)); // Output: charAt(0): console.log("concat(): " + text.concat(" Let's learn it.")); // Output: concat(): JavaScript is fun! Let's learn it. console.log("includes('fun'): " + text.includes("fun")); // Output: includes('fun'): true console.log("indexOf('S'): " + text.indexOf("S")); // Output: indexOf('S'): 5 console.log("replace('fun', 'awesome'): " + text.replace("fun", "awesome")); // Output: replace('fun', 'awesome'): JavaScript is awesome! console.log("slice(2, 12): " + text.slice(2, 12)); // Output: slice(2, 12): JavaScript console.log("split(' '): " + text.split(" ")); // Output: split(' '): ,JavaScript,is,fun!,, console.log("substring(2, 12): " + text.substring(2, 12)); // Output: substring(2, 12): JavaScript console.log("toLowerCase(): " + text.toLowerCase()); // Output: toLowerCase(): javascript is fun! console.log("toUpperCase(): " + text.toUpperCase()); // Output: toUpperCase(): JAVASCRIPT IS FUN! console.log("trim(): " + text.trim()); // Output: trim(): JavaScript is fun! </script> </body> </html>
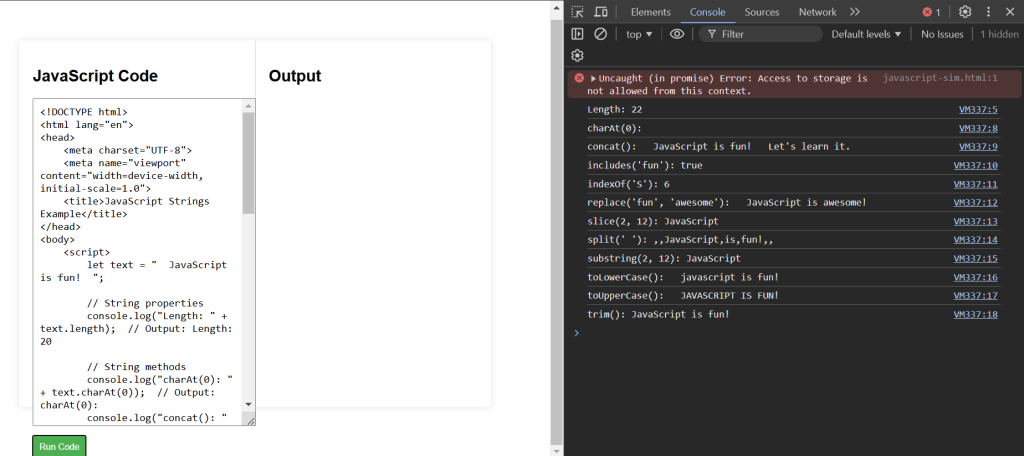