What are Events?
Events are actions or occurrences that happen in the browser, such as clicking a button, loading a page, or pressing a key. JavaScript can be used to listen for these events and execute code in response.
Common Event Types
- Mouse Events:
click
,dblclick
,mousedown
,mouseup
,mouseover
,mouseout
,mousemove
- Keyboard Events:
keydown
,keyup
,keypress
- Form Events:
submit
,reset
,focus
,blur
,change
- Window Events:
load
,resize
,scroll
,unload
Event Handlers
Event handlers are functions that execute when a specific event occurs. They can be assigned directly in HTML or using JavaScript.
Inline Event Handler
You can assign an event handler directly in an HTML element.
Explanation of Code:
When the button is clicked, the alert box displays the message “Button clicked!”.
<button onclick="alert('Button clicked!')">Click Me</button>
Adding Event Listeners
Using JavaScript, you can assign event handlers by adding event listeners. This approach separates JavaScript from HTML.
Explanation of Code:
An event listener is added to the button with the ID myButton
. When the button is clicked, the function displays an alert box.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Listeners Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
</script>
</body>
</html>
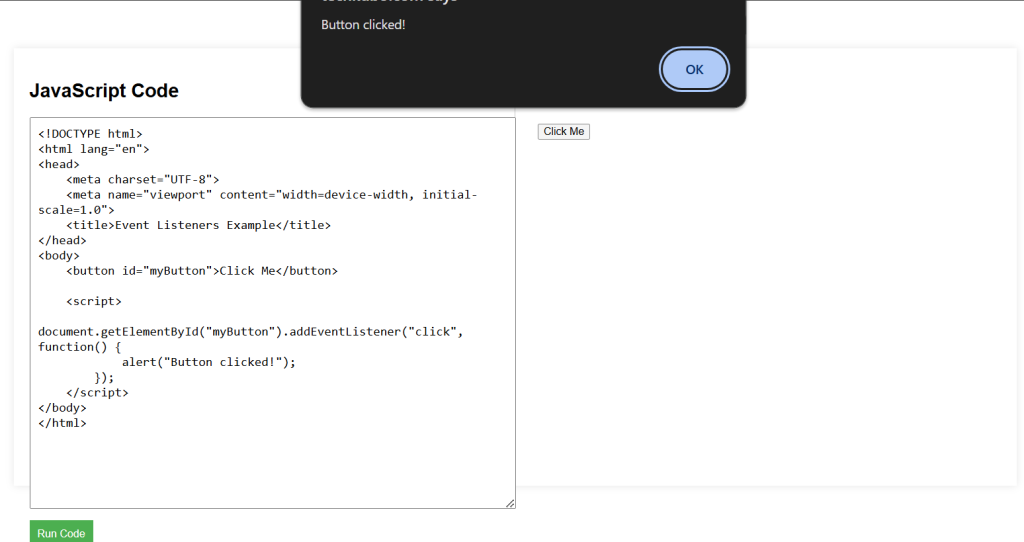
Removing Event Listeners
You can also remove event listeners using the removeEventListener
method.
Explanation of Code:
The event listener is removed, so clicking the button no longer triggers the alert.
let button = document.getElementById("myButton");
function showAlert() {
alert("Button clicked!");
}
button.addEventListener("click", showAlert);
// Removing the event listener
button.removeEventListener("click", showAlert);
Event Object
When an event occurs, an event object is automatically passed to the event handler. This object contains information about the event and its target element.
Explanation of Code:
The event object provides details about the event, such as its type and the target element that triggered it.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Object Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
document.getElementById("myButton").addEventListener("click", function(event) {
console.log("Event type: " + event.type);
console.log("Target element: " + event.target.tagName);
});
</script>
</body>
</html>
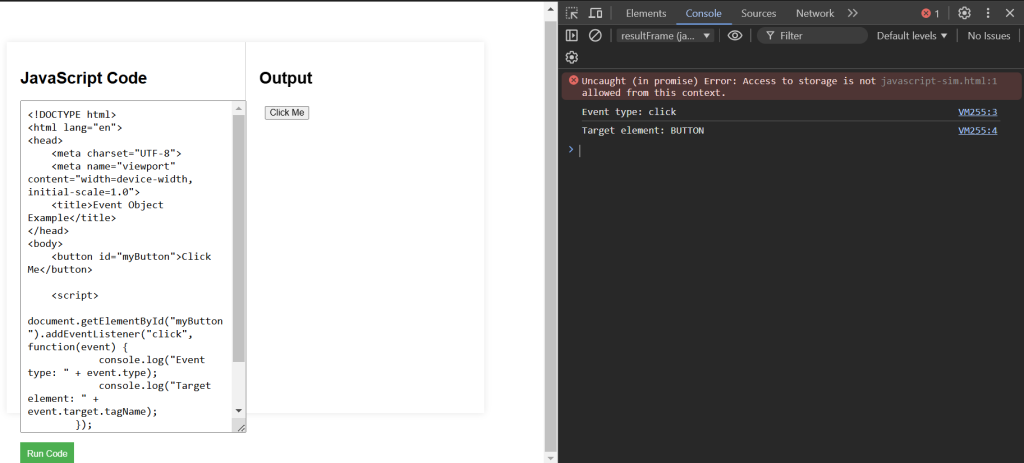
JavaScript Events Example Code
Explanation of Code:
- Mouse Events: Changes the color of the heading when the mouse hovers over and leaves it.
- Keyboard Events: Logs the key pressed in the console when typing in the input field.
- Form Events: Displays an alert with the input field’s value when the submit button is clicked, preventing the default form submission behavior.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Events Example</title>
</head>
<body>
<h1 id="heading">Move your mouse over me!</h1>
<input type="text" id="textInput" placeholder="Type something...">
<button id="submitButton">Submit</button>
<script>
let heading = document.getElementById("heading");
let textInput = document.getElementById("textInput");
let submitButton = document.getElementById("submitButton");
// Mouse event
heading.addEventListener("mouseover", function() {
heading.style.color = "blue";
});
heading.addEventListener("mouseout", function() {
heading.style.color = "black";
});
// Keyboard event
textInput.addEventListener("keydown", function(event) {
console.log("Key pressed: " + event.key);
});
// Form event
submitButton.addEventListener("click", function(event) {
event.preventDefault(); // Prevent the default form submission behavior
alert("Form submitted: " + textInput.value);
});
</script>
</body>
</html>
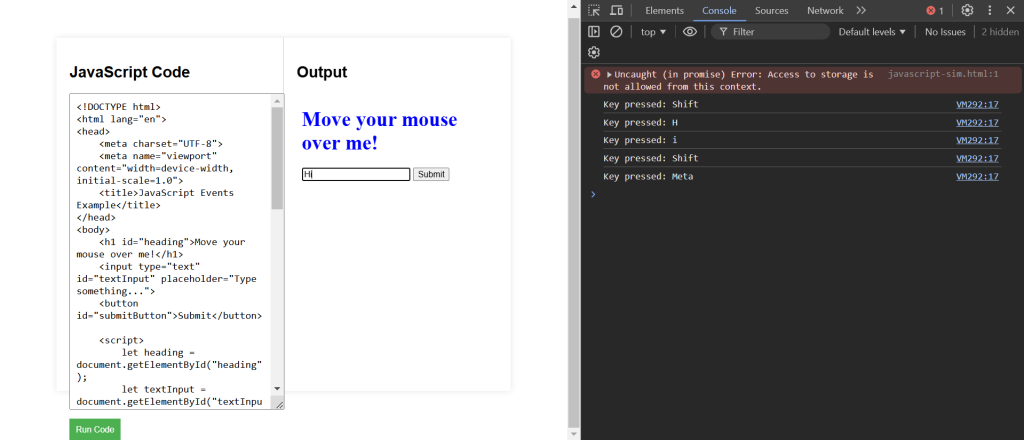