What is an Object?
An object is a collection of related data and functionalities, organized as properties and methods. Properties are values associated with an object, while methods are functions that perform actions on the object’s properties.
Creating an Object
You can create an object using object literal syntax, which involves defining the object within curly braces {}
with properties as key-value pairs.
Explanation of Code:
The person
object has properties firstName
, lastName
, age
, and isEmployed
with their respective values.
let person = {
firstName: "John",
lastName: "Doe",
age: 30,
isEmployed: true
};
console.log(person);
Accessing Object Properties
You can access the properties of an object using dot notation (object.property
) or bracket notation (object["property"]
).
Explanation of Code:
The firstName
property is accessed using dot notation, and the lastName
property is accessed using bracket notation.
console.log(person.firstName); // Output: John
console.log(person["lastName"]); // Output: Doe
Adding and Modifying Object Properties
You can add new properties to an object or modify existing ones using dot notation or bracket notation.
Explanation of Code:
A new property middleName
is added, and the age
property is modified.
person.middleName = "William";
person["age"] = 31;
console.log(person);
Deleting Object Properties
You can delete properties from an object using the delete
operator.
Explanation of Code:
The isEmployed
property is deleted from the person
object.
delete person.isEmployed;
console.log(person);
Methods
Methods are functions that are stored as properties of an object.
Explanation of Code:
The fullName
method concatenates the firstName
and lastName
properties to return the full name of the person.
let person = {
firstName: "John",
lastName: "Doe",
age: 30,
fullName: function() {
return this.firstName + " " + this.lastName;
}
};
console.log(person.fullName()); // Output: John Doe
JavaScript Objects Example Code
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Objects Example</title>
</head>
<body>
<script>
// Creating an object
let car = {
make: "Honda",
model: "Civic",
year: 2024,
color: "red",
start: function() {
return "Engine started";
},
stop: function() {
return "Engine stopped";
}
};
// Accessing properties
console.log("Make: " + car.make); // Output: Make: Honda
console.log("Model: " + car.model); // Output: Model: Civic
// Modifying properties
car.color = "Red";
console.log("Color: " + car.color); // Output: Color: Red
// Adding new properties
car.owner = "Josh";
console.log("Owner: " + car.owner); // Output: Owner: Josh
// Deleting properties
delete car.year;
console.log("Year: " + car.year); // Output: Year: undefined
// Using methods
console.log(car.start()); // Output: Engine started
console.log(car.stop()); // Output: Engine stopped
</script>
</body>
</html>
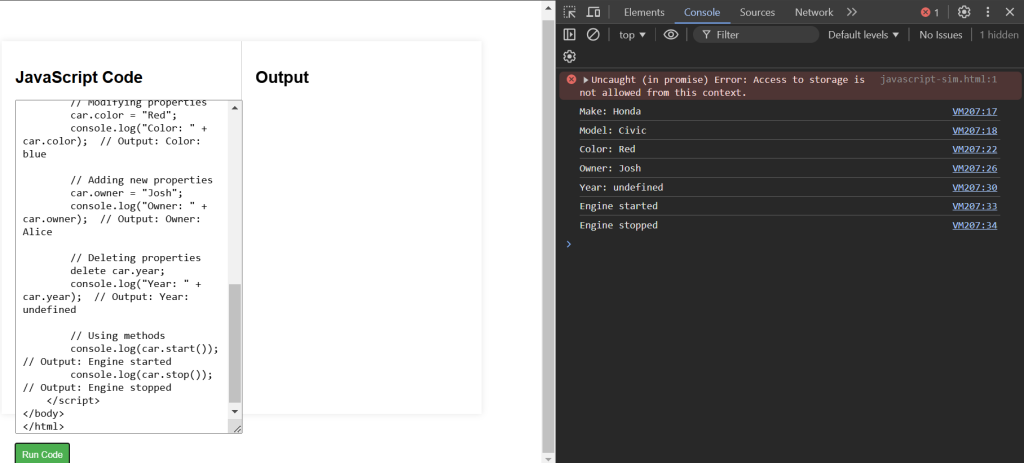