What are Variables?
Variables are used to store data that can be referenced and manipulated in a program. They act as containers for storing information. In JavaScript, you can declare variables using var
, let
, or const
.
Using var
The var
keyword is used to declare a variable, which can be reassigned later and has function scope.
JavaScript
x
var name = "John";
console.log(name); // Output: John
Using let
The let
keyword is used to declare a block-scoped variable, which can be reassigned.
JavaScript
let age = 30;
console.log(age); // Output: 30
Using const
The const
keyword is used to declare a block-scoped variable that cannot be reassigned.
JavaScript
const PI = 3.14;
console.log(PI); // Output: 3.14
Variable Naming Rules
- Variable names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter, underscore, or dollar sign.
- Variable names are case-sensitive (
myVar
andmyvar
are different). - Reserved words (like
let
,class
,return
, andfunction
) cannot be used as variable names.
Initializing Variables
Variables can be declared without being assigned a value. In such cases, they are initialized with the value undefined
.
JavaScript
let city;
console.log(city); // Output: undefined
city = "New York";
console.log(city); // Output: New York
Reassigning Variables
Variables declared with var
or let
can be reassigned new values.
JavaScript
let score = 50;
console.log(score); // Output: 50
score = 100;
console.log(score); // Output: 100
JavaScript Variables Example Code
Explanation of Code:
- Using
var
: Declares a variablename
and assigns it a value of “John”. - Using
let
: Declares a variableage
and assigns it a value of30
, which is later updated to31
. - Using
const
: Declares a constant variablePI
and assigns it a value of3.14
.
HTML
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Variables Example</title>
</head>
<body>
<script>
// Using var
var name = "John";
console.log("Name: " + name);
// Using let
let age = 30;
console.log("Age: " + age);
// Using const
const PI = 3.14;
console.log("PI: " + PI);
// Reassigning let variable
age = 31;
console.log("Updated Age: " + age);
</script>
</body>
</html>
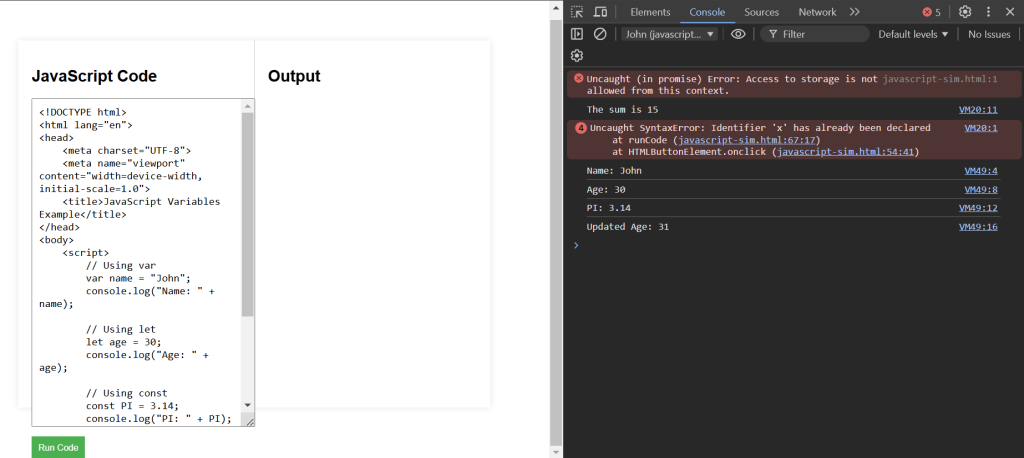