Single-Line Comments
Single-line comments are used to comment out a single line of code. They begin with //
.
Explanation of Code:
- The text following
//
is ignored by the JavaScript engine and is not executed as code.
JavaScript
x
// This is a single-line comment
let x = 5; // This is another single-line comment
Multi-Line Comments
Multi-line comments are used to comment out multiple lines of code. They begin with /*
and end with */
.
Explanation of Code:
- Everything between
/*
and*/
is ignored by the JavaScript engine.
JavaScript
/*
This is a multi-line comment
It can span multiple lines
*/
let y = 10;
Using Comments for Code Documentation
Comments are not just for temporarily disabling code. They are also used to explain the purpose of code, making it easier for others (or yourself) to understand it later.
Explanation of Code:
- Comments explain what the code does and the significance of each variable.
JavaScript
// Calculate the area of a rectangle
let width = 10; // Width of the rectangle
let height = 20; // Height of the rectangle
// Area is calculated as width multiplied by height
let area = width * height;
console.log("The area of the rectangle is " + area);
JavaScript Comments Example Code
Explanation of Code:
- Single-Line Comment: Used to describe the variable declaration.
- Multi-Line Comment: Used to explain the calculation.
- End-of-Line Comment: Used to document the output of the
console.log
statement.
HTML
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Comments Example</title>
</head>
<body>
<script>
// Single-line comment
let x = 5;
/*
Multi-line comment
explaining the calculation
*/
let y = 10;
let sum = x + y;
console.log("The sum is " + sum); // Output: The sum is 15
</script>
</body>
</html>
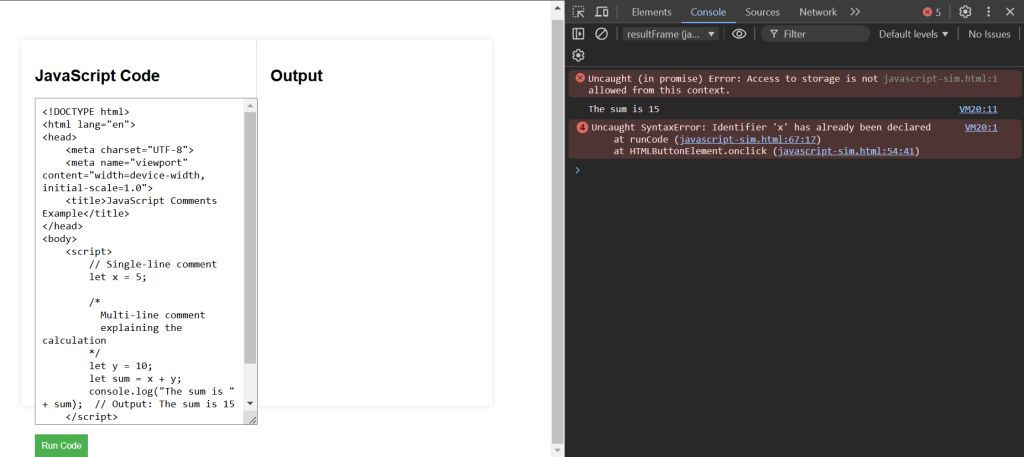