What are JavaScript Statements?
JavaScript statements are commands that tells the browser what to do. They are the building blocks of JavaScript code and are executed in the order they are written. Statements often end with a semicolon (;
), although it’s not always mandatory.
Assigment Statement
An assignment statement assigns a value to a variable.
JavaScript
x
let x = 10;
Function Call Statement
A function call statement invokes a function.
JavaScript
alert("Hello, World!");
Conditional Statement
A conditional statement executes code based on a condition.
JavaScript
if (x > 5) {
console.log("x is greater than 5");
}
Loop Statement
A loop statement repeats a block of code as long as a specified condition is true.
JavaScript
for (let i = 0; i < 5; i++) {
console.log("Number " + i);
}
JavaScript Code Blocks
A code block is a group of statements enclosed in curly braces {}
. It is often used with control structures like if
statements and loops to execute multiple statements.
JavaScript
{
let x = 10;
let y = 20;
let sum = x + y;
console.log("The sum is " + sum);
}
JavaScript Statements Example Code
Explanation of Code:
- Assignment Statement: Assigns the value
10
to the variablex
. - Function Call Statement: Displays an alert box with the value of
x
. - Conditional Statement: Checks if
x
is greater than5
and logs a message if true. - Loop Statement: Logs numbers
0
to4
to the console. - Code Block: Declares variables
y
andsum
, calculates the sum ofx
andy
, and logs the result.
JavaScript
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Statements Example</title>
</head>
<body>
<script>
// Assignment statement
let x = 10;
// Function call statement
alert("The value of x is " + x);
// Conditional statement
if (x > 5) {
console.log("x is greater than 5");
}
// Loop statement
for (let i = 0; i < 5; i++) {
console.log("Number " + i);
}
// Code block
{
let y = 20;
let sum = x + y;
console.log("The sum of x and y is " + sum);
}
</script>
</body>
</html>
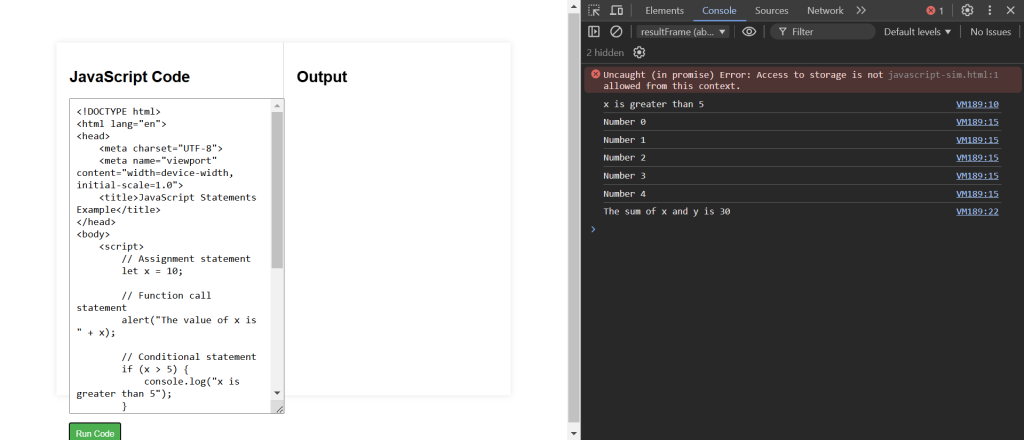