JavaScript Statements
JavaScript statements are instructions to be executed by the browser. They can perform actions like displaying output, making calculations, or manipulating the DOM. Each statement is usually separated by a semicolon (;
).
Explanation of Code:
Each line in the example above is a JavaScript statement. The last statement uses console.log
to output the value of z
.
let x = 5; let y = 6; let z = x + y; console.log(z); // Output: 11
Case Sensitivity
JavaScript is case-sensitive, meaning myVariable
and myvariable
are considered two different variables.
Explanation of Code:
The example demonstrates that myVariable
and myvariable
are treated as separate variables.
let myVariable = 5; let myvariable = 10; console.log(myVariable); // Output: 5 console.log(myvariable); // Output: 10
Whitespace and Indentation
JavaScript ignores extra spaces, tabs, and newlines, which means you can format your code for better readability without affecting its execution.
Exaplanation of Code:
The code uses indentation and spaces to make it more readable.
let a = 5; let b = 10; let c = a + b; console.log(c); // Output: 15
JavaScript Keywords
Keywords are reserved words that have special meaning in JavaScript. They are used to perform specific actions. Examples include var
, let
, const
, if
, else
, for
, while
, function
, and return
.
let name = "John"; // 'let' is a keyword used to declare variables if (name === "John") { console.log("Hello, John!"); // 'if' and 'else' are keywords used for conditional statements }
JavaScript Syntax Example Code
Explanation of Code:
- Variables: Declared using
let
. - Comments: Used both single-line and multi-line comments.
- Statements: Each statement ends with a semicolon.
- Output:
console.log
displays the result in the console.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Syntax Example</title> </head> <body> <script> // Declare variables let a = 5; let b = 10; /* Calculate the sum and display the result */ let sum = a + b; console.log("The sum is " + sum); // Output: The sum is 15 </script> </body> </html>
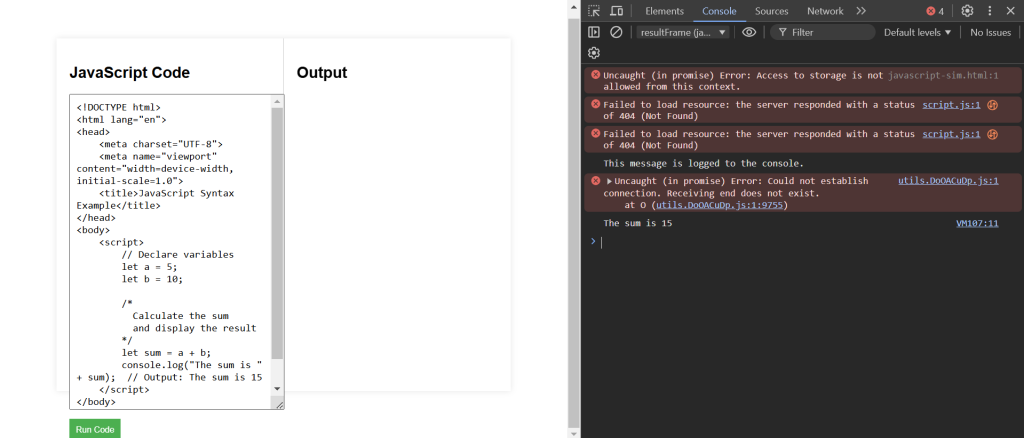