Using innerHTML
The innerHTML
property is used to change the HTML content of an element.
Explanation of Code:
The changeContent
function changes the content of the <p>
element with the ID demo
to “Content has been changed!” when the button is clicked.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript innerHTML Example</title> </head> <body> <p id="demo">This is a paragraph.</p> <button onclick="changeContent()">Change Content</button> <script> function changeContent() { document.getElementById("demo").innerHTML = "Content has been changed!"; } </script> </body> </html>
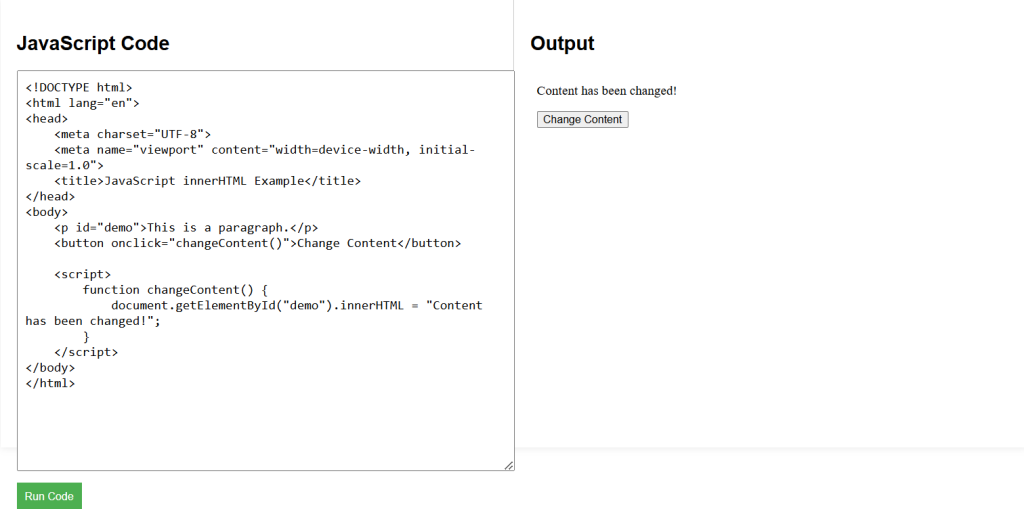
Using document.write()
The document.write()
method writes directly to the HTML document. It’s typically used for testing purposes and not recommended for production.
Explanation of Code:
The document.write
method writes the text “This is written directly to the document.” to the HTML document.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript document.write Example</title> </head> <body> <script> document.write("This is written directly to the document."); </script> </body> </html>
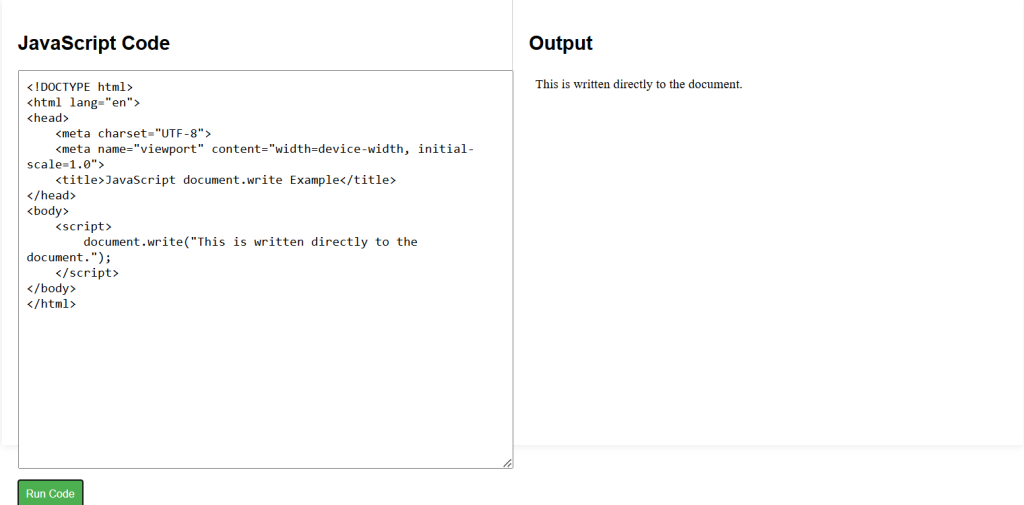
Using alert()
The alert()
method displays a message in an alert box.
Explanation of Code:
The showAlert
function displays an alert box with the message “This is an alert box!” when the button is clicked.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript alert Example</title> </head> <body> <button onclick="showAlert()">Show Alert</button> <script> function showAlert() { alert("This is an alert box!"); } </script> </body> </html>
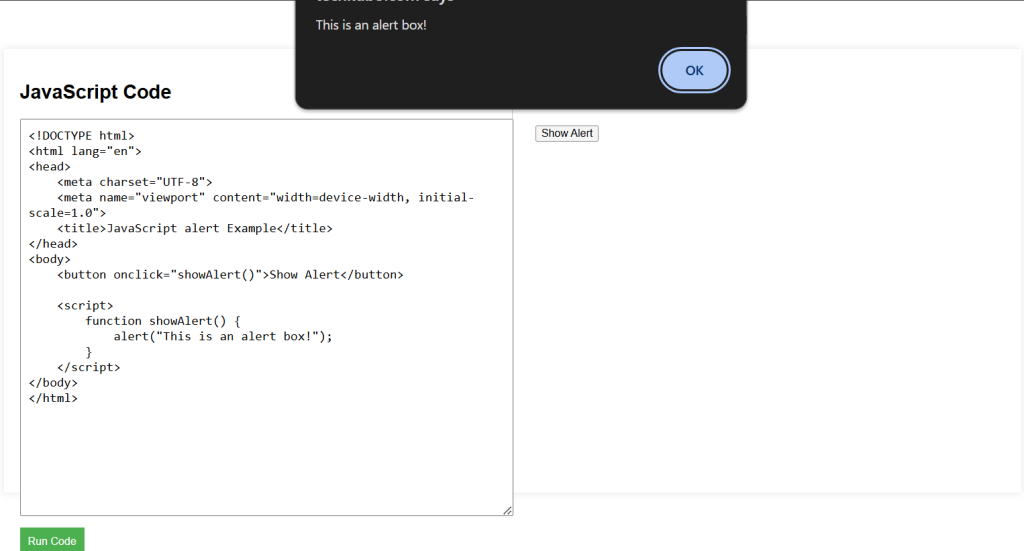
Using console.log()
The console.log()
method is used to display output in the browser’s console, which is useful for debugging.
Explanation of Code:
The console.log
method logs the message “This message is logged to the console.” to the browser’s console.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript console.log Example</title> </head> <body> <script> console.log("This message is logged to the console."); </script> </body> </html>
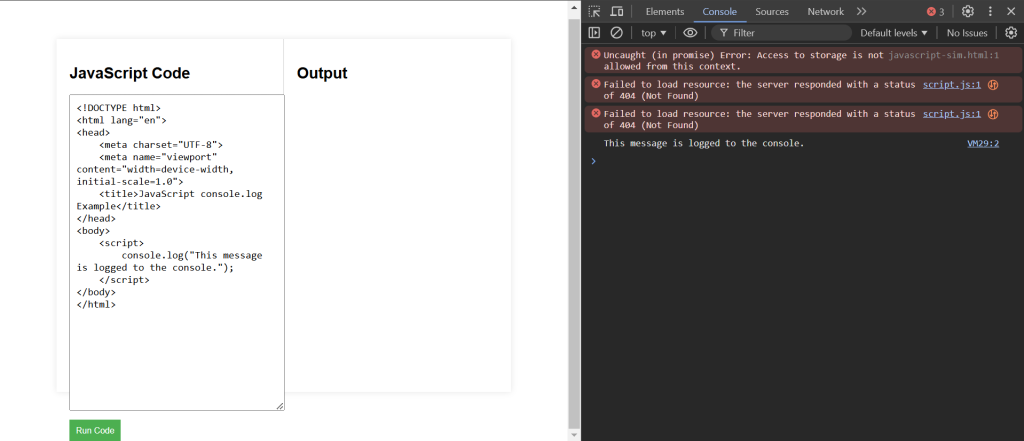