Inline JavaScript
Inline JavaScript is written directly within an HTML element’s attribute. This method is typically used for small snippets of code.
Explanation of Code:
In this example, the JavaScript code is placed within the onclick
attribute of the <button>
element. When the button is clicked, an alert box displays the message “Hello, World!”.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Inline JavaScript Example</title> </head> <body> <button onclick="alert('Hello, World!')">Click Me</button> </body> </html>
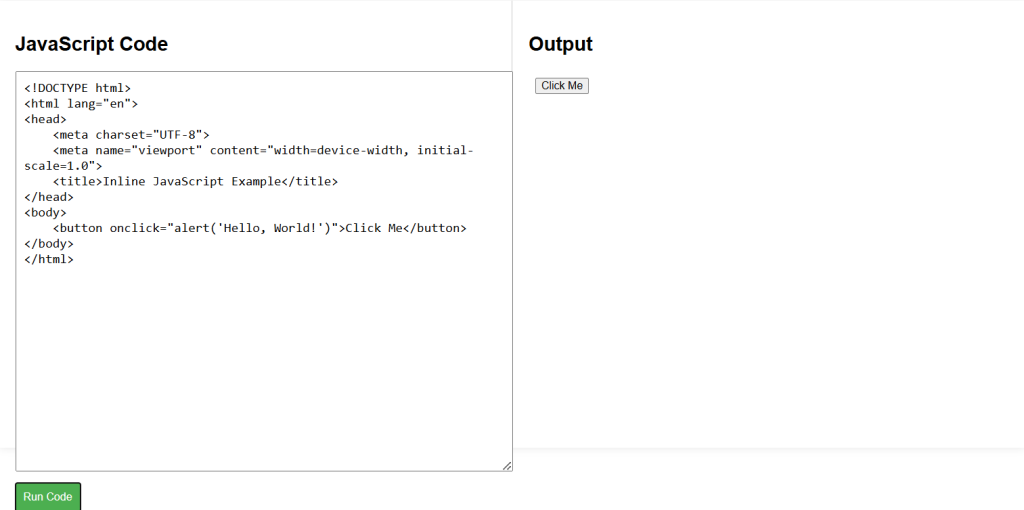
Internal JavaScript
Internal JavaScript is written within the <script>
tag, inside the HTML file. It can be placed in the <head>
or <body>
section of the document.
Explanation of Code:
The JavaScript code is placed inside a <script>
tag within the <head>
section. The function showMessage
is defined, and it is called when the button is clicked.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Internal JavaScript Example</title> <script> function showMessage() { alert('Hello, World!'); } </script> </head> <body> <button onclick="showMessage()">Click Me</button> </body> </html>
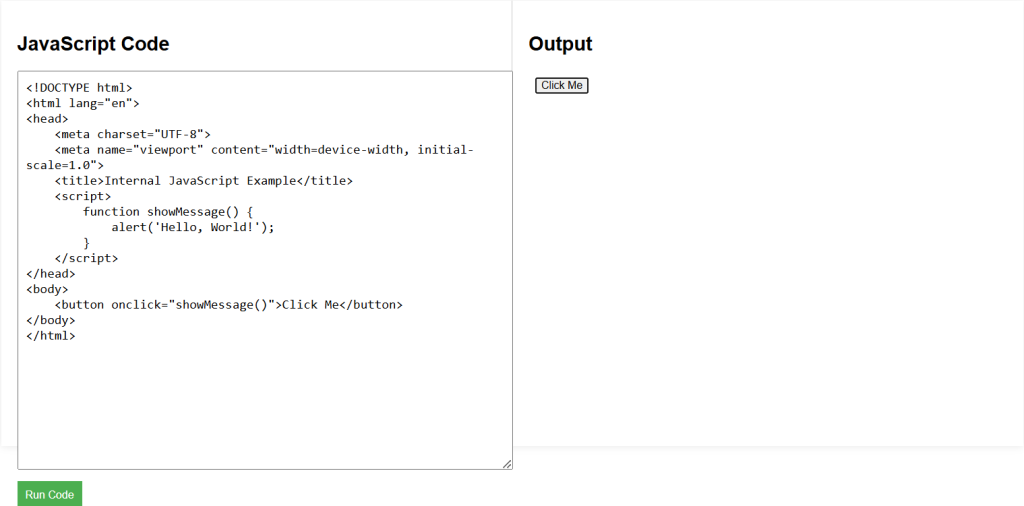
External JavaScript
External JavaScript is written in a separate .js
file, which is then linked to the HTML document. This method keeps the HTML and JavaScript code separate, making the code easier to maintain.
Explanation of Code:
The JavaScript code is written in an external file named script.js
. The <script>
tag with the src
attribute is used to include the external JavaScript file in the HTML document. The function showMessage
is defined in the external file and is called when the button is clicked.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>External JavaScript Example</title> <script src="script.js"></script> </head> <body> <button onclick="showMessage()">Click Me</button> </body> </html>
function showMessage() { alert('Hello, World!'); }
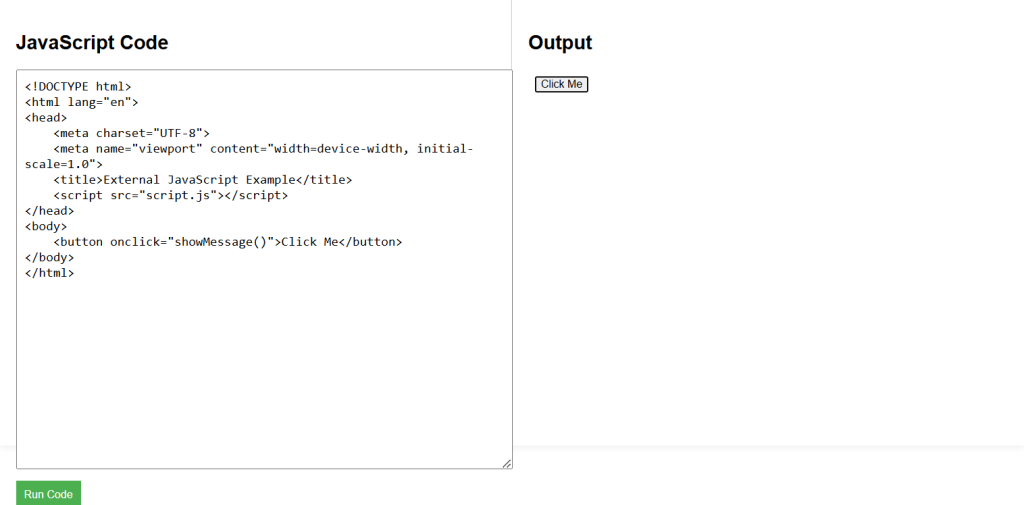