The switch
statement is another way to control the flow of your code based on different conditions. It can be more concise than multiple if-else
statements when dealing with multiple possible values for a single variable.
Switch Statement Syntax
The switch
statement evaluates an expression and matches the expression’s value to a case
label. If a match is found, the associated block of code is executed.
Explanation of Code:
The switch
statement evaluates expression
and executes the code corresponding to the matching case
label. The break
statement is used to exit the switch block. The default
case is executed if no match is found.
switch (expression) { case value1: // code to be executed if expression === value1 break; case value2: // code to be executed if expression === value2 break; // additional cases here default: // code to be executed if expression doesn't match any case }
JavaScript Switch Example Code
Explanation of Code:
This HTML file sets up a dropdown menu where users can select a fruit. The JavaScript code uses a switch
statement to display a message based on the selected fruit.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Switch Statement Example</title> </head> <body> <h1>Switch Statement Example</h1> <label for="fruit">Choose a fruit:</label> <select id="fruit" onchange="showFruitMessage()"> <option value="apple">Apple</option> <option value="banana">Banana</option> <option value="cherry">Cherry</option> <option value="date">Date</option> </select> <p id="fruitMessage"></p> <script> function showFruitMessage() { let fruit = document.getElementById('fruit').value; let message = document.getElementById('fruitMessage'); switch (fruit) { case 'apple': message.textContent = "Apples are red or green."; break; case 'banana': message.textContent = "Bananas are yellow."; break; case 'cherry': message.textContent = "Cherries are small and red."; break; case 'date': message.textContent = "Dates are sweet and brown."; break; default: message.textContent = "Please select a fruit."; } } </script> </body> </html>
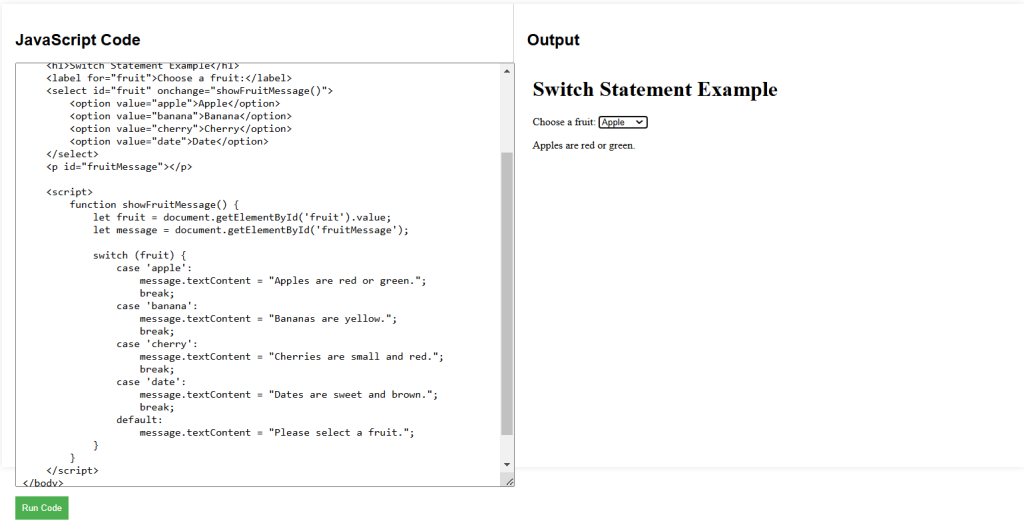