If Statement
The if
statement executes a block of code if a specified condition is true.
Explanation of Code:
This code checks if the number is greater than 5. If the condition is true, it executes the code inside the block.
let number = 10;
if (number > 5) {
console.log("The number is greater than 5");
}
If-Else Statement
The if-else
statement executes one block of code if a condition is true and another block of code if the condition is false.
Explanation of Code:
This code checks if the number is greater than 5. If true, it executes the first block; otherwise, it executes the second block.
let number = 3;
if (number > 5) {
console.log("The number is greater than 5");
} else {
console.log("The number is 5 or less");
}
Else If Statement
The else if
statement allows you to test multiple conditions.
Explanation of Code:
This code checks multiple conditions and executes the corresponding block of code based on which condition is true.
let number = 5;
if (number > 5) {
console.log("The number is greater than 5");
} else if (number === 5) {
console.log("The number is exactly 5");
} else {
console.log("The number is less than 5");
}
Ternary Operator
The ternary operator is a shorthand for the if-else
statement. It takes three operands: a condition, an expression to execute if the condition is true, and an expression to execute if the condition is false.
Explanation of Code:
This code uses the ternary operator to check if the number is greater than 5 and assigns the appropriate message to the result
variable.
let number = 10;
let result = (number > 5) ? "Greater than 5" : "5 or less";
console.log(result); // "Greater than 5"
JavaScript Conditionals Example Code
Explanation of Code:
This HTML file sets up an age verification form. The JavaScript code checks the user’s input age and displays a message indicating whether the user is an adult, a minor, or if the input is invalid.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Age Verification</title>
</head>
<body>
<h1>Age Verification</h1>
<input type="number" id="ageInput" placeholder="Enter your age">
<button onclick="verifyAge()">Verify Age</button>
<p id="ageResult"></p>
<script>
function verifyAge() {
let age = parseInt(document.getElementById('ageInput').value);
let result = document.getElementById('ageResult');
if (age >= 18) {
result.textContent = "You are an adult.";
} else if (age > 0 && age < 18) {
result.textContent = "You are a minor.";
} else {
result.textContent = "Please enter a valid age.";
}
}
</script>
</body>
</html>
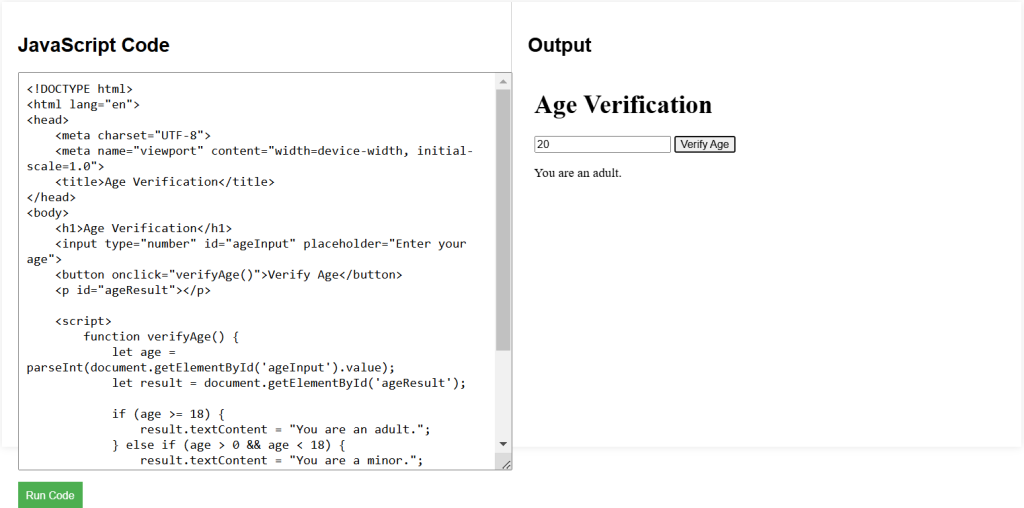