Comparison operators in JavaScript allow you to compare two values and determine the relationship between them. These comparisons result in boolean values (true
or false
), making them essential for controlling the flow of your code with conditional statements.
Common Comparison Operators
- Equality (
==
):
Explanation of Code:
The double equals operator checks if two values are equal, allowing type conversion if necessary.
let isEqual = (5 == '5'); // true
- Strict Equality (
===
):
Explanation of Code:
The triple equals operator checks if two values are equal without type conversion.
let isStrictEqual = (5 === '5'); // false
- Inequality (
!=
):
Explanation of Code:
The not equals operator checks if two values are not equal, allowing type conversion if necessary.
let isNotEqual = (5 != '5'); // false
- Strict Inequality (
!==
):
Explanation of Code:
The strict not equals operator checks if two values are not equal without type conversion.
let isStrictNotEqual = (5 !== '5'); // true
- Greater Than (
>
):
Explanation of Code:
The greater than operator checks if the left operand is greater than the right operand.
let isGreaterThan = (10 > 5); // true
- Less Than (
<
):
Explanation of Code:
The less than operator checks if the left operand is less than the right operand.
let isLessThan = (5 < 10); // true
- Greater Than or Equal To (
>=
):
Explanation of Code:
The greater than or equal to operator checks if the left operand is greater than or equal to the right operand.
let isGreaterOrEqual = (10 >= 10); // true
- Less Than or Equal To (
<=
):
Explanation of Code:
The less than or equal to operator checks if the left operand is less than or equal to the right operand.
let isLessOrEqual = (5 <= 10); // true
JavaScript Comparisons Example Code
Explanation of Code:
This HTML file sets up a simple form where users can input a number. The JavaScript code compares the input number with 10 and displays an appropriate message.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Comparison Operators Example</title> </head> <body> <h1>Comparison Operators Example</h1> <input type="text" id="numberInput" placeholder="Enter a number"> <button onclick="compareNumber()">Compare Number</button> <p id="comparisonResult"></p> <script> function compareNumber() { let number = parseInt(document.getElementById('numberInput').value); let result = document.getElementById('comparisonResult'); if (number > 10) { result.textContent = "The number is greater than 10."; } else if (number === 10) { result.textContent = "The number is exactly 10."; } else { result.textContent = "The number is less than 10."; } } </script> </body> </html>
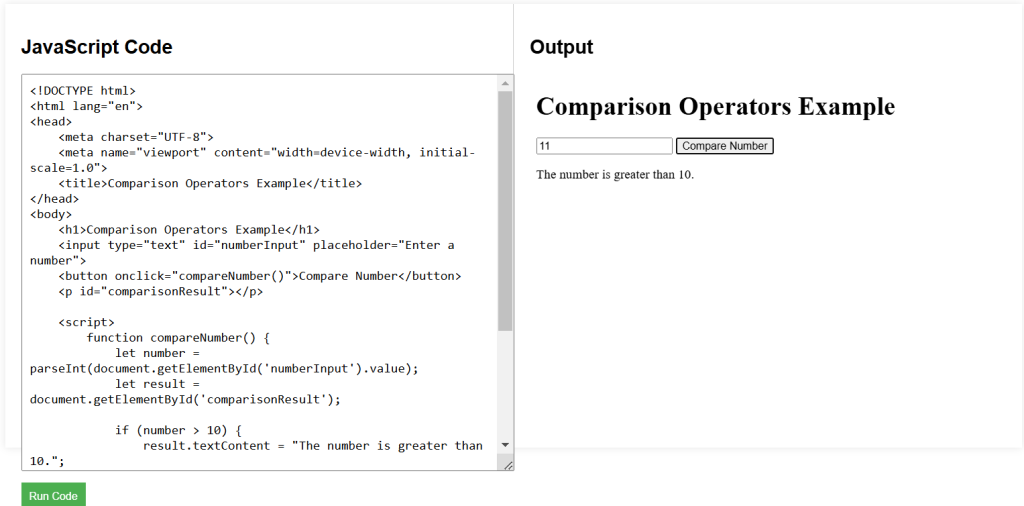