Booleans represent one of the simplest data types in JavaScript. They can hold only two values: true
or false
. Booleans are often used in conditional statements to control the flow of a program.
Boolean Values
You can declare boolean variables in JavaScript using the keywords true
and false
.
- Declaring Boolean Variables:
Explanation of Code:
Here, isJavaScriptFun
is set to true
and isFishMammal
is set to false
.
let isJavaScriptFun = true; let isFishMammal = false;
Logical Operators
Logical operators are used to combine multiple boolean expressions.
- Logical AND (
&&
):
Explanation of Code:
The &&
operator returns true
only if both operands are true
.
let andResult = (true && false); // false
- Logical OR (
||
):
Explanation of Code:
The ||
operator returns true
if at least one of the operands is true
.
let orResult = (true || false); // true
- Logical NOT (
!
):
Explanation of Code:
The !
operator negates the boolean value of its operand.
let notResult = !true; // false
JavaScript Boolean Example Code
Explanation of Code:
This HTML file sets up a simple form where users can input a number. The JavaScript code checks if the number is greater than 10 and displays an appropriate message.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Boolean Operators Example</title> </head> <body> <h1>Boolean Operators Example</h1> <form> <label for="number1">Enter the first number:</label> <input type="number" id="number1" required><br><br> <label for="number2">Enter the second number:</label> <input type="number" id="number2" required><br><br> <button type="button" onclick="compareNumbers()">Compare</button> </form> <p id="comparisonResult"></p> <script> function compareNumbers() { let num1 = parseFloat(document.getElementById('number1').value); let num2 = parseFloat(document.getElementById('number2').value); let result = document.getElementById('comparisonResult'); let areEqual = (num1 === num2); let notEqual = (num1 !== num2); let greaterThan = (num1 > num2); let lessThan = (num1 < num2); let greaterOrEqual = (num1 >= num2); let lessOrEqual = (num1 <= num2); result.innerHTML = ` Are the numbers equal? ${areEqual}<br> Are the numbers not equal? ${notEqual}<br> Is the first number greater than the second? ${greaterThan}<br> Is the first number less than the second? ${lessThan}<br> Is the first number greater than or equal to the second? ${greaterOrEqual}<br> Is the first number less than or equal to the second? ${lessOrEqual} `; } </script> </body> </html>
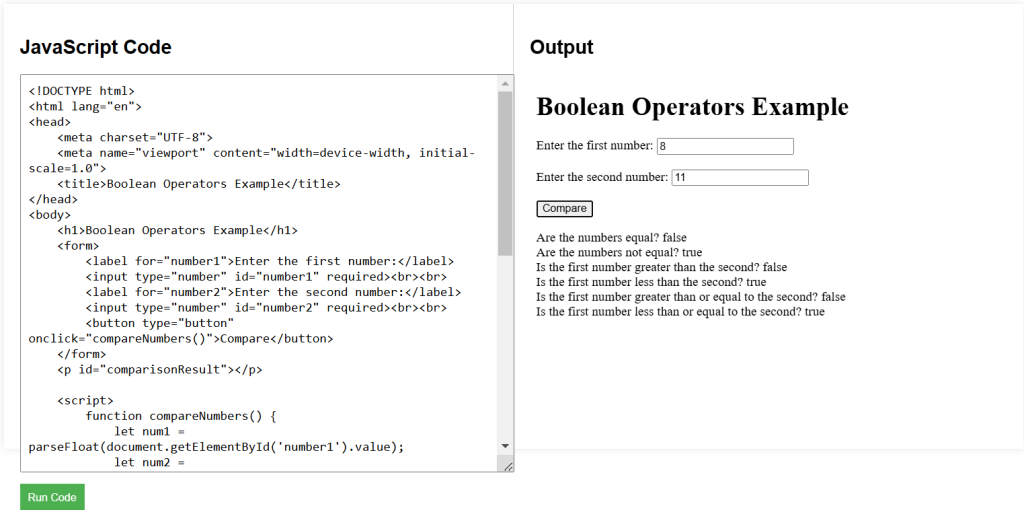