JavaScript provides the Math.random()
method to generate random numbers. This method returns a floating-point number between 0 (inclusive) and 1 (exclusive).
Generating Random Numbers
- Math.random():
Explanation of Code:
This method returns a random floating-point number between 0 (inclusive) and 1 (exclusive).
let randomNum = Math.random(); // Random number between 0 and 1
Generating Random Integers
- Random Integer between 0 and 10:
Explanation of Code:
This code generates a random integer between 0 and 10 by multiplying the result of Math.random()
by 11 and using Math.floor()
to round down to the nearest integer.
let randomInt = Math.floor(Math.random() * 11); // Random integer between 0 and 10
- Random Integer between Two Values:
Explanation of Code:
This function generates a random integer between the specified min
and max
values. It uses Math.ceil()
to round min
up, Math.floor()
to round max
down, and then calculates the random integer.
function getRandomInt(min, max) { min = Math.ceil(min); max = Math.floor(max); return Math.floor(Math.random() * (max - min + 1)) + min; } let randomInt = getRandomInt(5, 15); // Random integer between 5 and 15
JavaScript Random Example Code
Explanation of Code:
This HTML file sets up a random color generator. When you click the “Generate Color” button, it generates a random RGB color and updates the background color of the page.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Random Color Generator</title> </head> <body> <h1>Random Color Generator</h1> <button onclick="generateRandomColor()">Generate Color</button> <p id="colorDisplay"></p> <script> function getRandomInt(min, max) { min = Math.ceil(min); max = Math.floor(max); return Math.floor(Math.random() * (max - min + 1)) + min; } function generateRandomColor() { let r = getRandomInt(0, 255); let g = getRandomInt(0, 255); let b = getRandomInt(0, 255); let color = `rgb(${r}, ${g}, ${b})`; document.getElementById('colorDisplay').textContent = `Random Color: ${color}`; document.body.style.backgroundColor = color; } </script> </body> </html>
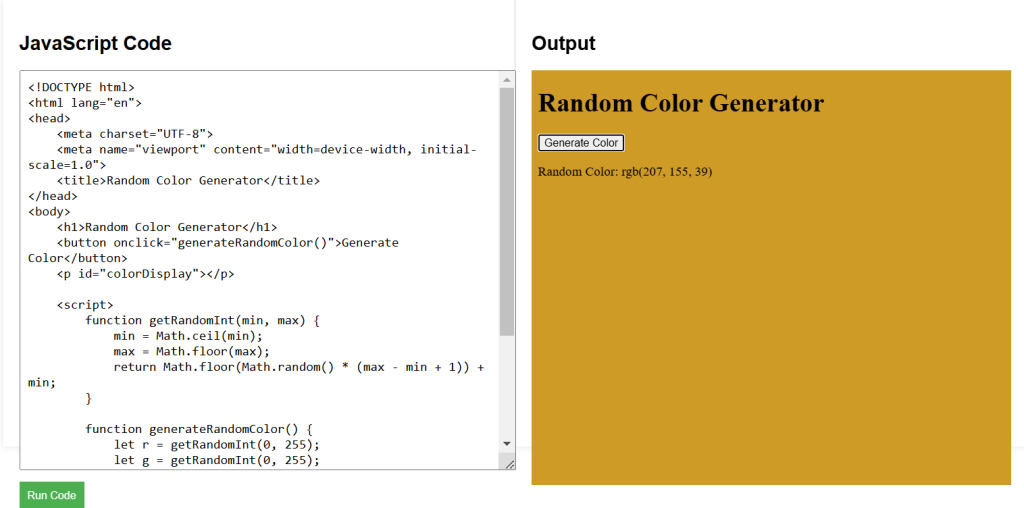