Common Math Methods
- Math.abs():
Explanation of Code:
Returns the absolute value of a number.
let absoluteValue = Math.abs(-7.5); // 7.5
- Math.ceil():
Explanation of Code:
Rounds a number up to the nearest integer.
let roundedUp = Math.ceil(4.2); // 5
- Math.floor():
Explanation of Code:
Rounds a number down to the nearest integer.
let roundedDown = Math.floor(4.9); // 4
- Math.round():
Explanation: of Code:
Rounds a number to the nearest integer.
let rounded = Math.round(4.5); // 5
- Math.max():
Explanation of Code:
Returns the largest of the given numbers.
let maximum = Math.max(1, 2, 3, 4, 5); // 5
- Math.min():
Explanation of Code:
Returns the smallest of the given numbers.
let minimum = Math.min(1, 2, 3, 4, 5); // 1
- Math.random():
Explanation of Code:
Returns a random number between 0 (inclusive) and 1 (exclusive).
let randomNum = Math.random(); // Random number between 0 and 1
- Math.sqrt():
Explanation of Code:
Returns the square root of a number.
let squareRoot = Math.sqrt(25); // 5
JavaScript Math Example Code
Explanation of Code:
This HTML file sets up a random integer generator that displays a random integer between 1 and 100.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Random Integer Generator</title> </head> <body> <h1>Random Integer Generator</h1> <p id="randomInteger"></p> <script> function getRandomInt(min, max) { min = Math.ceil(min); max = Math.floor(max); return Math.floor(Math.random() * (max - min + 1)) + min; } let randomInteger = getRandomInt(1, 100); document.getElementById('randomInteger').textContent = `Random integer between 1 and 100: ${randomInteger}`; </script> </body> </html>
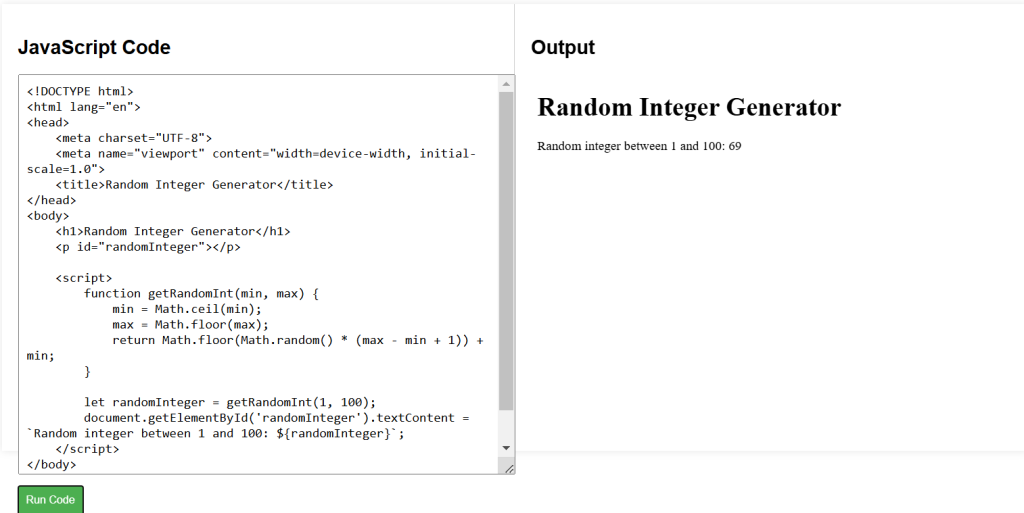