What is an Array?
An array is a special variable that can hold multiple values at once. Arrays are useful for storing lists of data, such as numbers, strings, or objects.
Creating Arrays
You can create arrays using the array literal syntax or the Array
constructor.
Array Literal Syntax
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits); // Output: ["Apple", "Banana", "Cherry"]
Array Constructor
let fruits = new Array("Apple", "Banana", "Cherry");
console.log(fruits); // Output: ["Apple", "Banana", "Cherry"]
Accessing Array Elements
You can access array elements using their index. Array indices start at 0.
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits[0]); // Output: Apple
console.log(fruits[1]); // Output: Banana
console.log(fruits[2]); // Output: Cherry
Modifying Array Elements
You can modify elements in an array by accessing them using their index.
let fruits = ["Apple", "Banana", "Cherry"];
fruits[1] = "Blueberry";
console.log(fruits); // Output: ["Apple", "Blueberry", "Cherry"]
Array Properties
Length
The length
property returns the number of elements in an array.
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits.length); // Output: 3
Array Methods
JavaScript provides various methods to manipulate arrays.
1. push()
The push()
method adds one or more elements to the end of an array.
let fruits = ["Apple", "Banana"];
fruits.push("Cherry");
console.log(fruits); // Output: ["Apple", "Banana", "Cherry"]
2. pop()
The pop()
method removes the last element from an array.
let fruits = ["Apple", "Banana", "Cherry"];
fruits.pop();
console.log(fruits); // Output: ["Apple", "Banana"]
3. shift()
The shift()
method removes the first element from an array.
let fruits = ["Apple", "Banana", "Cherry"];
fruits.shift();
console.log(fruits); // Output: ["Banana", "Cherry"]
4. unshift()
The unshift()
method adds one or more elements to the beginning of an array.
let fruits = ["Banana", "Cherry"];
fruits.unshift("Apple");
console.log(fruits); // Output: ["Apple", "Banana", "Cherry"]
5. splice()
The splice()
method adds or removes elements from an array.
let fruits = ["Apple", "Banana", "Cherry"];
fruits.splice(1, 1, "Blueberry", "Mango");
console.log(fruits); // Output: ["Apple", "Blueberry", "Mango", "Cherry"]
6. slice()
The slice()
method returns a new array containing a portion of an existing array.
let fruits = ["Apple", "Banana", "Cherry"];
let citrus = fruits.slice(1, 3);
console.log(citrus); // Output: ["Banana", "Cherry"]
7. concat()
The concat()
method merges two or more arrays.
let fruits = ["Apple", "Banana"];
let moreFruits = ["Cherry", "Date"];
let allFruits = fruits.concat(moreFruits);
console.log(allFruits); // Output: ["Apple", "Banana", "Cherry", "Date"]
8. join()
The join()
method joins all elements of an array into a string.
let fruits = ["Apple", "Banana", "Cherry"];
let fruitString = fruits.join(", ");
console.log(fruitString); // Output: "Apple, Banana, Cherry"
JavaScript Arrays Example Code
Explanation of Code:
- Creating Arrays: Demonstrates creating arrays using the array literal syntax.
- Accessing Elements: Accesses elements using their index.
- Modifying Elements: Modifies elements using their index.
- Array Properties: Uses the
length
property to get the number of elements. - Array Methods: Demonstrates various array methods such as
push
,pop
,shift
,unshift
,splice
,slice
,concat
, andjoin
.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Arrays Example</title>
</head>
<body>
<script>
let fruits = ["Apple", "Banana", "Cherry"];
// Accessing elements
console.log("First fruit: " + fruits[0]); // Output: First fruit: Apple
// Modifying elements
fruits[1] = "Blueberry";
console.log("Modified fruits: " + fruits); // Output: Modified fruits: Apple,Blueberry,Cherry
// Array properties
console.log("Number of fruits: " + fruits.length); // Output: Number of fruits: 3
// Array methods
fruits.push("Mango");
console.log("After push: " + fruits); // Output: After push: Apple,Blueberry,Cherry,Mango
fruits.pop();
console.log("After pop: " + fruits); // Output: After pop: Apple,Blueberry,Cherry
fruits.shift();
console.log("After shift: " + fruits); // Output: After shift: Blueberry,Cherry
fruits.unshift("Apple");
console.log("After unshift: " + fruits); // Output: After unshift: Apple,Blueberry,Cherry
fruits.splice(1, 1, "Pineapple", "Grapes");
console.log("After splice: " + fruits); // Output: After splice: Apple,Pineapple,Grapes,Cherry
let citrus = fruits.slice(1, 3);
console.log("After slice: " + citrus); // Output: After slice: Pineapple,Grapes
let moreFruits = ["Orange", "Peach"];
let allFruits = fruits.concat(moreFruits);
console.log("After concat: " + allFruits); // Output: After concat: Apple,Pineapple,Grapes,Cherry,Orange,Peach
let fruitString = fruits.join(", ");
console.log("After join: " + fruitString); // Output: After join: Apple, Pineapple, Grapes, Cherry
</script>
</body>
</html>
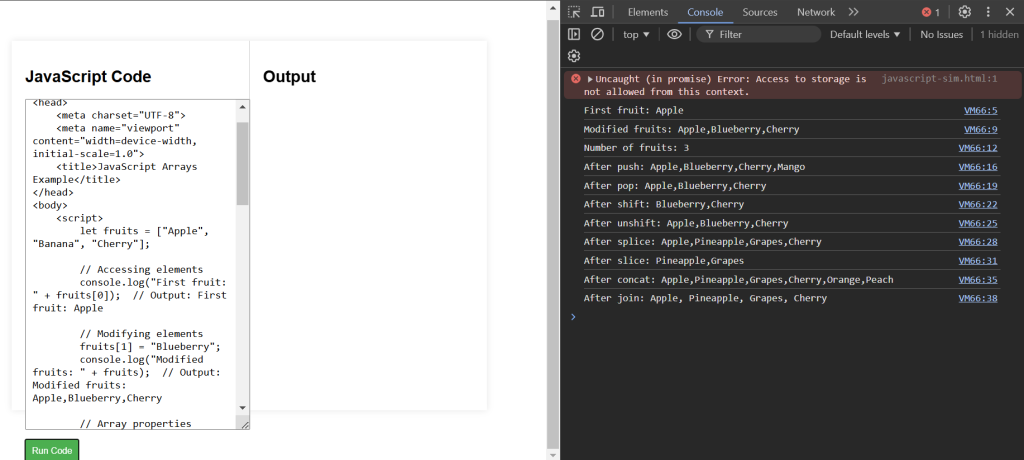