1. Static Positioning (Default)
By default, all elements have position: static
. This means they follow the normal flow of the document without any special positioning. Static positioning doesn’t allow you to move elements from their default positions on the page. It simply follows the natural flow of the content.
div { position: static; /* default behavior */ }
2. Relative Positioning
When you use position: relative
, the element stays in the normal document flow, but you can move it relative to its original position using the top
, bottom
, left
, or right
properties.
div { position: relative; top: 20px; /* Moves the element 20px down */ left: 15px; /* Moves the element 15px to the right */ }
3. Absolute Positioning
With position: absolute
, the element is removed from the document flow and positioned relative to its nearest positioned ancestor (an element with relative
, absolute
, or fixed
positioning).
div { position: absolute; top: 30px; right: 10px; }
4. Fixed Positioning
An element with position: fixed
is positioned relative to the viewport (browser window), meaning it stays in the same place even when you scroll.
div { position: fixed; bottom: 0; right: 0; }
5. Z-Index and Stacking Order
The z-index
property controls the stacking order of elements, allowing you to determine which element should appear on top when elements overlap. The higher the z-index
value, the higher the element’s position in the stack.
div { position: absolute; z-index: 10; }
CSS Positioning Example Code
Explanation of Code:
- Static: Follows normal document flow.
- Relative: Moves relative to its original position but keeps its space in the document.
- Absolute: Positioned based on its nearest positioned ancestor.
- Fixed: Stays in place even while scrolling.
- Z-Index: Controls which element appears on top when they overlap.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>CSS Positioning Example</title> <style> .static-box { position: static; background-color: lightblue; padding: 10px; margin-bottom: 10px; border: 2px solid blue; width: 100%; } .relative-box { position: relative; top: 20px; background-color: lightgreen; padding: 10px; margin-bottom: 10px; border: 2px solid green; width: 100%; } .absolute-box { position: absolute; top: 150px; left: 20px; background-color: lightcoral; padding: 10px; width: 80%; border: 2px solid red; } .fixed-box { position: fixed; bottom: 10px; right: 10px; background-color: lightgray; padding: 10px; width: 200px; border: 2px solid gray; } .z-index-high { position: absolute; top: 180px; left: 30px; background-color: yellow; padding: 10px; z-index: 10; border: 2px solid orange; } .z-index-low { position: absolute; top: 200px; left: 50px; background-color: pink; padding: 10px; z-index: 1; border: 2px solid purple; } </style> </head> <body> <div class="static-box">This is a static element (default positioning).</div> <div class="relative-box">This is a relative element (moved 20px from its original position).</div> <div class="absolute-box">This is an absolute element (positioned 150px from the top and 20px from the left).</div> <div class="z-index-high">This is a high z-index (above the pink box).</div> <div class="z-index-low">This is a lower z-index (below the yellow box).</div> <div class="fixed-box">This is a fixed element (always stays at the bottom-right corner).</div> </body> </html>
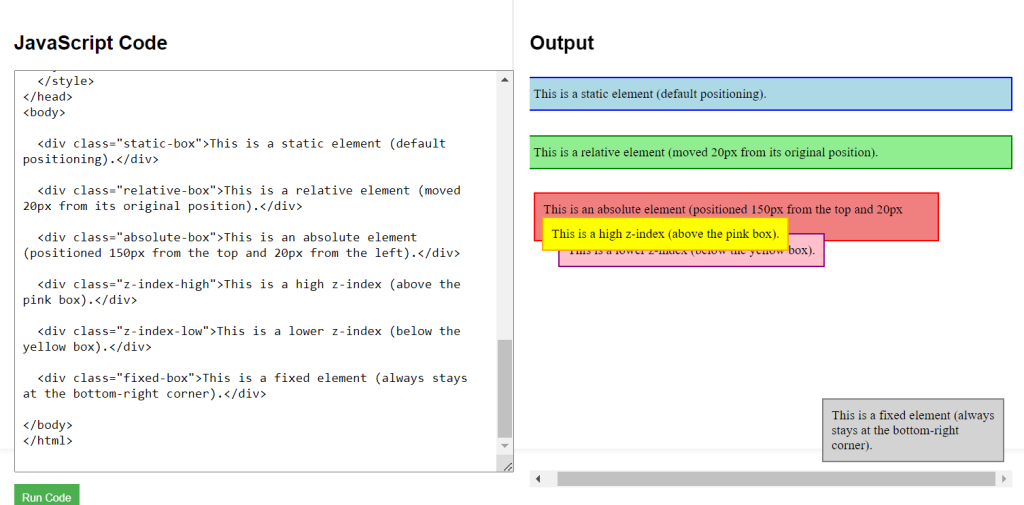