The display
Property
The display
property determines how an element is displayed in the document. Here are some commonly used values:
block
: Displays the element as a block-level element. It starts on a new line and takes up the full width available.
CSS
x
div {
display: block;
}
inline
: Displays the element as an inline element. It doesn’t start on a new line, and it only takes up as much width as necessary.
CSS
span {
display: inline;
}
inline-block
: Similar toinline
, but you can set width and height properties.
CSS
div {
display: inline-block;
}
none
: The element is completely removed from the document flow and is not visible on the page.
CSS
div {
display: none;
}
CSS Display Example Code
HTML
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Display Property Example</title>
<style>
.block-element {
display: block;
width: 100%;
background-color: lightblue;
padding: 10px;
margin-bottom: 10px;
}
.inline-element {
display: inline;
background-color: lightgreen;
padding: 10px;
}
.inline-block-element {
display: inline-block;
background-color: lightcoral;
width: 200px;
padding: 10px;
margin: 5px;
}
.none-element {
display: none;
}
</style>
</head>
<body>
<div class="block-element">This is a block element. It takes up the full width available.</div>
<span class="inline-element">This is an inline element.</span>
<span class="inline-element">This is also an inline element.</span>
<div class="inline-block-element">This is an inline-block element. It behaves like an inline element but can have width and height.</div>
<div class="inline-block-element">Another inline-block element.</div>
<div class="none-element">This element will not be displayed.</div>
</body>
</html>
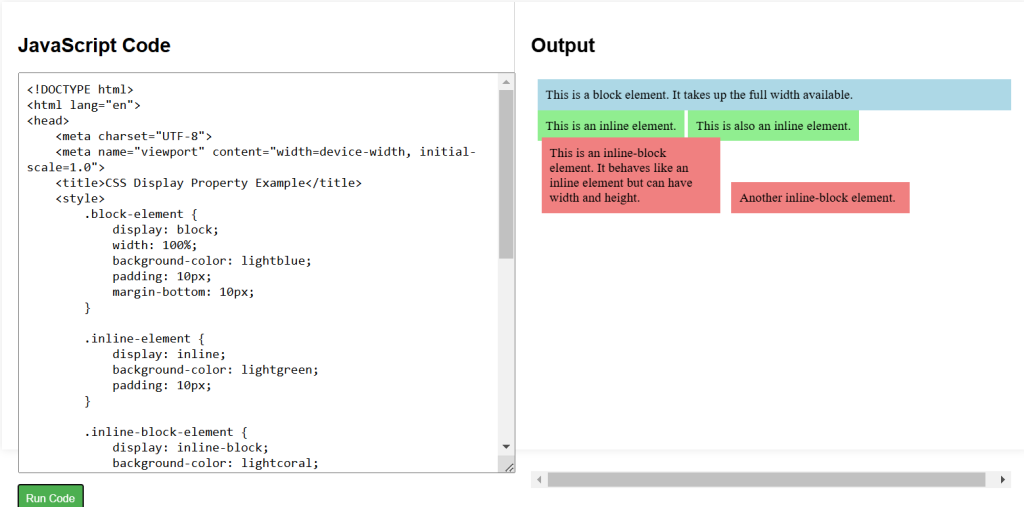