1. Background Color
The background-color
property sets the background color of an element. You can use color names, HEX codes, RGB, RGBA, HSL, or HSLA values to define the color.
div { background-color: lightblue; }
You can also apply transparency using RGBA or HSLA:
div { background-color: rgba(0, 0, 255, 0.3); /* Semi-transparent blue */ }
2. Background Image
To use an image as the background of an element, you can apply the background-image
property. Make sure to specify the image URL.
div { background-image: url('background.jpg'); }
3. Background Repeat
By default, a background image repeats itself to fill the element. The background-repeat
property allows you to control this behavior.
repeat
: The default behavior, repeating the image both horizontally and vertically.no-repeat
: Prevents the image from repeating.repeat-x
: Repeats the image only horizontally.repeat-y
: Repeats the image only vertically.
div { background-image: url('background.jpg'); background-repeat: no-repeat; }
4. Background Position
The background-position
property sets the starting position of the background image. You can define it using keywords (e.g., top
, center
, bottom
) or coordinates (e.g., 50% 50%
).
div { background-image: url('background.jpg'); background-position: center; }
Coordinates allow more precise positioning:
div { background-position: 100px 50px; /* 100px from the left, 50px from the top */ }
5. Background Size
The background-size
property controls the size of the background image. You can specify the size using pixels, percentages, or keywords.
cover
: Scales the image to cover the entire element (may crop parts of the image).contain
: Scales the image to fit inside the element (may leave empty space).
div { background-image: url('background.jpg'); background-size: cover; }
You can also define custom sizes:
div { background-image: url('background.jpg'); background-size: 300px 200px; }
6. Background Attatchment
The background-attachment
property controls whether the background scrolls with the page or remains fixed.
scroll
: The background moves when the user scrolls the page (default).fixed
: The background stays fixed in place.local
: The background scrolls with the content inside the element.
div { background-image: url('background.jpg'); background-attachment: fixed; }
7. Background Gradient
CSS also allows you to create gradients as backgrounds using the linear-gradient
and radial-gradient
functions.
- Linear Gradient:
/*This creates a background that transitions from red to yellow horizontally.*/ div { background: linear-gradient(to right, red, yellow); }
- Radial Gradient:
/*This creates a circular gradient starting from red and transitioning to yellow.*/ div { background: radial-gradient(circle, red, yellow); }
8. Shorthand for Background Properties
You can combine multiple background properties into one shorthand declaration. This shorthand sets the background image, no-repeat, center position, and cover size all in one line.
div { background: url('background.jpg') no-repeat center/cover; }
CSS Backgrounds Example Code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { background-color: #f0f0f0; } .background-example { width: 300px; height: 200px; background-image: url('background.jpg'); background-size: cover; background-position: center; background-attachment: fixed; border: 2px solid black; padding: 20px; margin: 20px auto; text-align: center; } </style> <title>CSS Backgrounds Example</title> </head> <body> <div class="background-example"> This box has a background image with various properties applied. </div> </body> </html
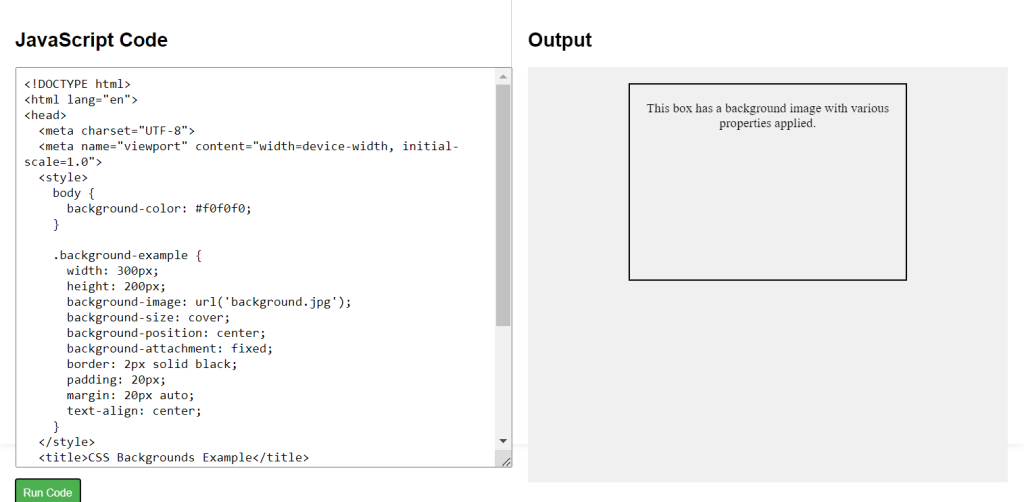