What is the CSS Box Model
Every element in CSS is a rectangular box. The CSS Box Model defines how the size of this box is calculated, including the content, padding, border, and margin. Understanding the Box Model is crucial for designing the layout of a web page, as it determines how elements are sized and spaced.
The four components of the Box Model are:
- Content: The actual content of the box (e.g., text or images).
- Padding: Space between the content and the border.
- Border: A line that surrounds the padding (if any) and the content.
- Margin: Space outside the border between this box and other elements.
+------------+ Margin | | | +------+ | Border | | | | | | Text | | Padding | | | | | +------+ | Content | | +------------+
Content Area
The content is where your text, images, or other elements live. This area can be sized using properties like width
and height
.
div { width: 200px; height: 100px; background-color: lightblue; }
In this example, the div
will have a width of 200 pixels and a height of 100 pixels. The content will fit inside this space.
Padding
The padding is the space between the content and the border of an element. You can control padding on all sides or individually for top, right, bottom, and left.
div { padding: 20px; }
This adds 20 pixels of space around the content inside the div
.
If you want to set padding for specific sides:
div { padding-top: 10px; padding-right: 15px; padding-bottom: 20px; padding-left: 25px; }
Or shorthand:
div { padding: 10px 15px 20px 25px; /* top, right, bottom, left */ }
Border
The border goes around the padding and content. You can set the width, style, and color of the border.
div { border: 2px solid black; }
In this example, the border is 2 pixels wide, solid, and black. You can also set borders individually for each side:
div { border-top: 5px dashed red; border-right: 3px solid blue; }
Margin
The margin is the space outside the border. It defines the distance between this element and neighboring elements. You can set margins just like padding:
div { margin: 20px; }
Or specify margins for each side individually:
div { margin-top: 10px; margin-right: 15px; margin-bottom: 20px; margin-left: 25px; }
Shorthand for margins follows the same pattern as padding:
div { margin: 10px 15px 20px 25px; /* top, right, bottom, left */ }
Box-Sizing Property
By default, the width and height of an element only apply to the content area. Padding and borders are added to that width and height, potentially making the element larger than expected. You can change this behavior using the box-sizing
property.
div { box-sizing: border-box; }
With box-sizing: border-box
, the padding and borders are included in the width and height, making layout calculations easier.
CSS Box Model Example Code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> div { width: 300px; padding: 20px; border: 5px solid black; margin: 30px; background-color: lightblue; } </style> <title>CSS Box Model Example</title> </head> <body> <div> This box has a content area, padding, border, and margin. The total width includes all these components. </div> </body> </html>
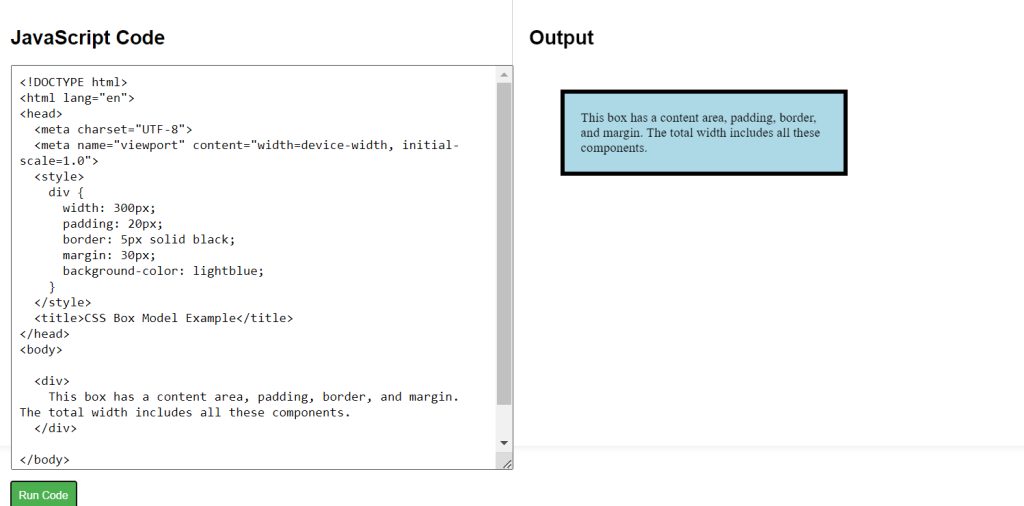