CSS selectors are used to target HTML elements and apply styles to them.
Element Selector
Targets elements by their tag name.
CSS
x
p {
color: blue;
}
Class Selector
Targets elements with a specific class attribute, using a dot ( . ).
CSS
.example {
font-weight: bold;
}
ID Selector
Targets an element with a specific ID attribute, using a hastag ( # ).
CSS
#unique {
font-style: italic;
}
Universal Selector
Targets all elements on the page.
CSS
* {
margin: 0;
padding: 0;
}
Group Selector
Applies the same styles to multiple selectors, separated by commas.
CSS
h1, h2, h3 {
color: purple;
}
Descendant Selector
Targets elements that are descendants of another element.
CSS
div p {
color: green;
}
Child Selector
Targets direct children of a specified element.
CSS
ul > li {
list-style: none;
}
Adjacent Sibling Selector
Targets an element immediately following a specified element.
CSS
h1 + p {
margin-top: 0;
}
General Sibling Selector
Targets all sibling elements following a specified element.
CSS
h1 ~ p {
color: red;
}
Attribute Selector
Targets elements based on their attribute values.
CSS
a[target="_blank"] {
color: orange;
}
CSS Selector Example Code
HTML
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Selectors Example</title>
<style>
/* Element selector */
p {
color: blue;
}
/* Class selector */
.highlight {
background-color: yellow;
}
/* ID selector */
#special {
font-weight: bold;
}
/* Universal selector */
* {
margin: 0;
padding: 0;
}
/* Group selector */
h1, h2, h3 {
color: purple;
}
/* Descendant selector */
div p {
color: green;
}
/* Child selector */
ul > li {
list-style: none;
}
/* Adjacent sibling selector */
h1 + p {
margin-top: 0;
}
/* General sibling selector */
h1 ~ p {
color: red;
}
/* Attribute selector */
a[target="_blank"] {
color: orange;
}
</style>
</head>
<body>
<h1>Heading 1</h1>
<h2>Heading 2</h2>
<h3>Heading 3</h3>
<p>This is a paragraph.</p>
<div>
<p>This is a paragraph inside a div.</p>
</div>
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<p class="highlight">This is a highlighted paragraph.</p>
<p id="special">This is a special paragraph.</p>
<a href="https://example.com" target="_blank">Visit Example</a>
</body>
</html>
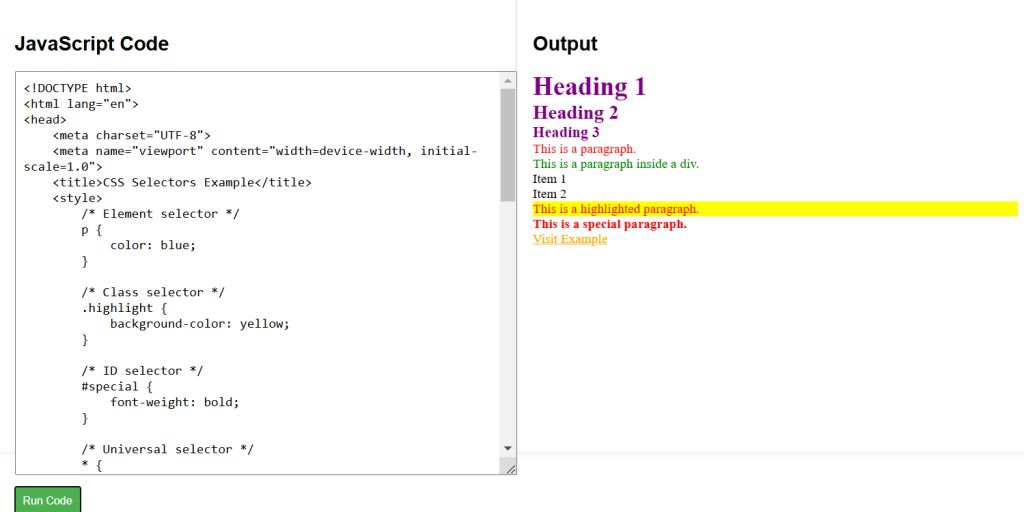