The <template>
element is a powerful feature in HTML that allows you to declare fragments of HTML that are not rendered immediately but can be instantiated later using JavaScript.
HTML Template Syntax
Explanation of Syntax:
<template>
: The element that defines the template. It will not be displayed in the document until it is instantiated.id="template-id"
: An optional attribute to uniquely identify the template for future reference.
<template id="template-id"> <div> <h2>Title</h2> <p>Description</p> </div> </template>
HTML Template Example Code
Explanation of Code:
<template>
Element: This contains the structure for each item. It’s defined once and can be reused multiple times.id="item-template"
: This ID allows us to easily reference the template in our JavaScript.items
Array: This array holds the data we want to display. Each object represents an item with a title and description.- JavaScript Logic:
- The template is retrieved using
document.getElementById()
. - For each item in the
items
array, a clone of the template’s content is created usingdocument.importNode()
. - The title and description are then filled in using
textContent
. - Finally, the populated clone is appended to the
#item-container
element, making the items visible in the document.
- The template is retrieved using
<template id="item-template"> <div class="item"> <h3 class="item-title"></h3> <p class="item-description"></p> </div> </template> <div id="item-container"></div> <script> const template = document.getElementById('item-template'); const container = document.getElementById('item-container'); const items = [ { title: "Item 1", description: "This is the first item." }, { title: "Item 2", description: "This is the second item." }, { title: "Item 3", description: "This is the third item." }, ]; items.forEach(item => { const clone = document.importNode(template.content, true); clone.querySelector('.item-title').textContent = item.title; clone.querySelector('.item-description').textContent = item.description; container.appendChild(clone); }); </script>
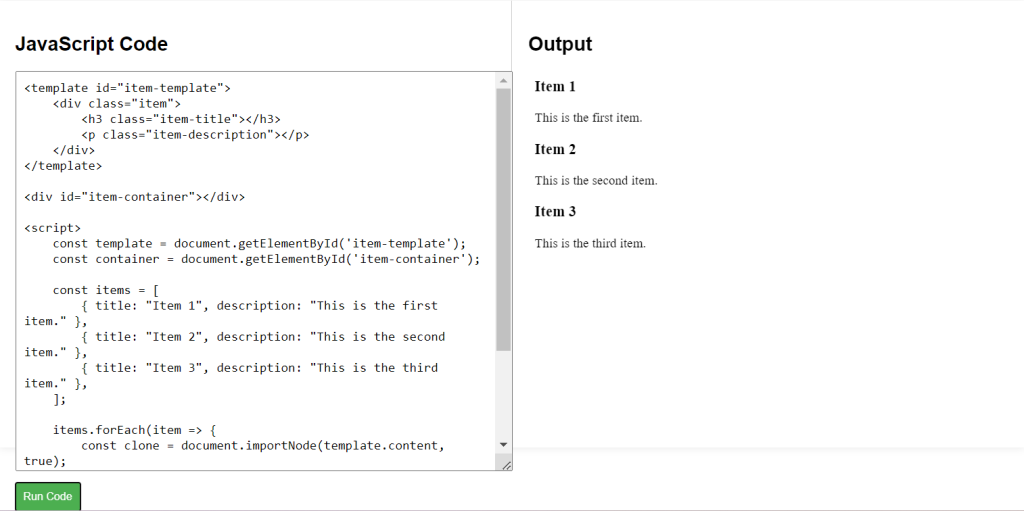